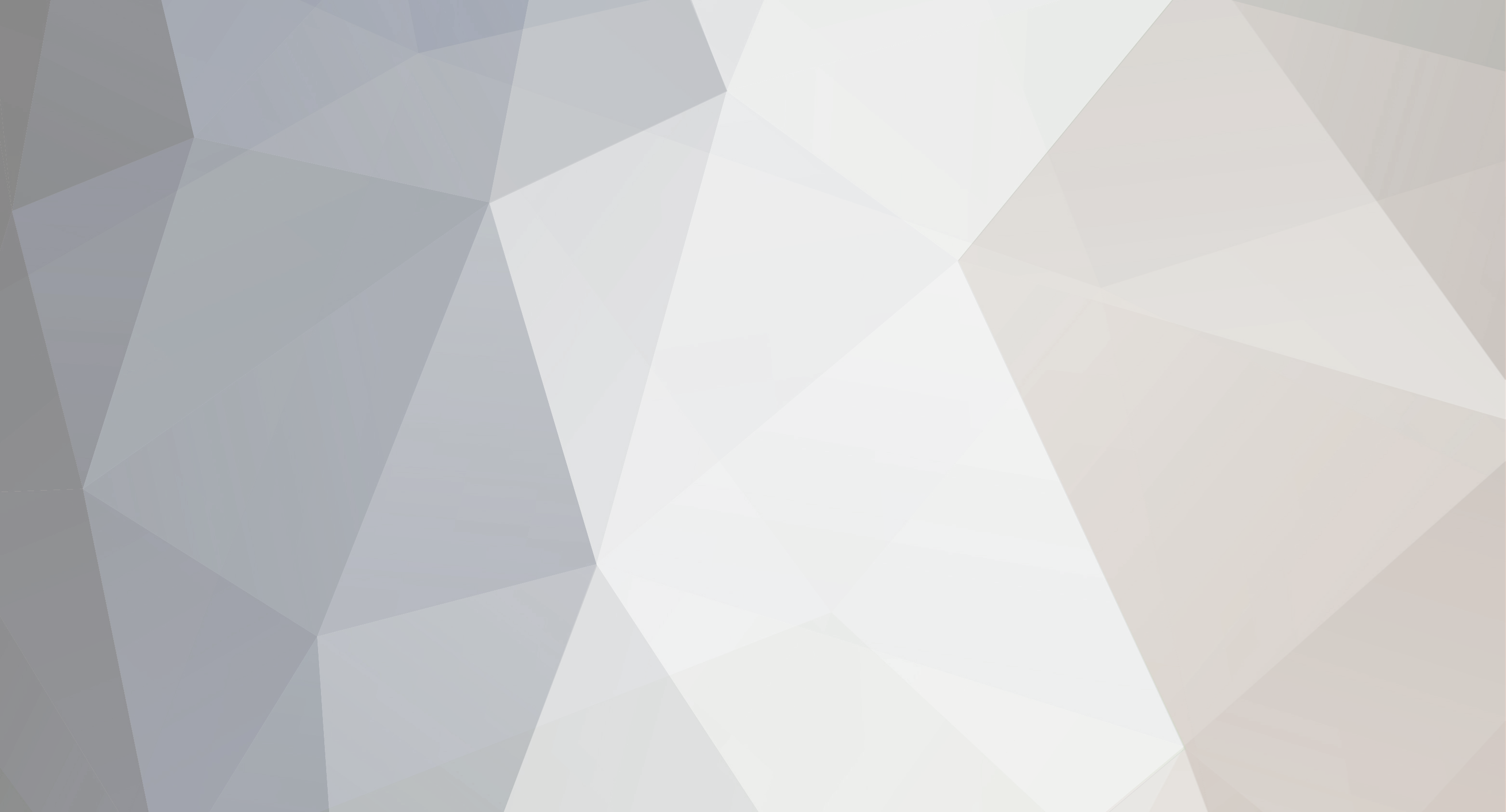
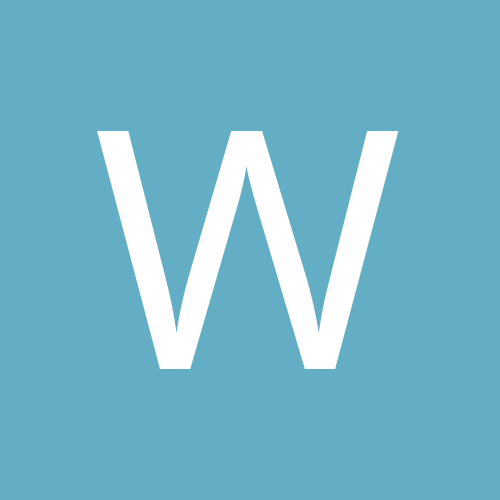
WRieder
-
Content Count
8 -
Joined
-
Last visited
Posts posted by WRieder
-
-
Attached is the code for the complete project
-
I started with trying to run it from the Delphi IDE with
PythonEngine1.ExecStrings(SynEdit1.Lines);
but get an error
Project Project1.exe raised exception class EPyException with message 'error: OpenCV(4.6.0) D:\a\opencv-python\opencv-python\opencv_contrib\modules\face\src\facerec.cpp:61: error: (-2:Unspecified error) File can't be opened for reading! in function 'cv::face::FaceRecognizer::read''.
It runs perfectly from the command prompt as well as in PyScripter.
I thought about doing something similar to Demo29
-
Thank you for replying so quickly Below the code: Real Time Face Recogition ==> Each face stored on dataset/ dir, should have a unique numeric integer ID as 1, 2, 3, etc ==> LBPH computed model (trained faces) should be on trainer/ dir Based on original code by Anirban Kar: https://github.com/thecodacus/Face-Recognition Developed by Marcelo Rovai - MJRoBot.org @ 21Feb18 ''' import cv2 import io import numpy as np import os from PIL import Image from io import BytesIO import face_recognition import sys recognizer = cv2.face.LBPHFaceRecognizer_create() recognizer.read('trainer/trainer.yml') cascadePath = "haarcascade_frontalface_default.xml" faceCascade = cv2.CascadeClassifier(cascadePath); font = cv2.FONT_HERSHEY_SIMPLEX #iniciate id counter id = 0 # names related to ids: example ==> Marcelo: id=1, etc names = ['None', 'Wolfgang', 'Bruno', 'Devlyn', 'Z', 'W'] # Initialize and start realtime video capture cam = cv2.VideoCapture(0) cam.set(3, 640) # set video widht cam.set(4, 480) # set video height # Define min window size to be recognized as a face minW = 0.15*cam.get(3) minH = 0.15*cam.get(4) while True: ret, img =cam.read() gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY) faces = faceCascade.detectMultiScale( gray, scaleFactor = 1.2, minNeighbors = 5, minSize = (int(minW), int(minH)), ) for(x,y,w,h) in faces: cv2.rectangle(img, (x,y), (x+w,y+h), (0,255,0), 2) id, confidence = recognizer.predict(gray[y:y+h,x:x+w]) # Check if confidence is less them 100 ==> "0" is perfect match if (confidence < 100): id = names[id] confidence = " {0}%".format(round(100 - confidence)) else: id = "unknown" confidence = " {0}%".format(round(100 - confidence)) cv2.putText(img, str(id), (x+5,y-5), font, 1, (255,255,255), 2) cv2.putText(img, str(confidence), (x+5,y+h-5), font, 1, (255,255,0), 1) cv2.imshow('camera',img) stream = BytesIO(img) stream.getvalue() k = cv2.waitKey(10) & 0xff # Press 'ESC' for exiting video if k == 27: break # Do a bit of cleanup print("\n [INFO] Exiting Program and cleanup stuff") cam.release() cv2.destroyAllWindows()
-
A script, which works perfectly in PyScripter gives an error when executed in Delphi:
'''' Real Time Face Recogition ==> Each face stored on dataset/ dir, should have a unique numeric integer ID as 1, 2, 3, etc ==> LBPH computed model (trained faces) should be on trainer/ dir Based on original code by Anirban Kar: https://github.com/thecodacus/Face-Recognition Developed by Marcelo Rovai - MJRoBot.org @ 21Feb18 ''' import cv2 import numpy as np import os recognizer = cv2.face.LBPHFaceRecognizer_create() recognizer.read('trainer/trainer.yml') cascadePath = "haarcascade_frontalface_default.xml" faceCascade = cv2.CascadeClassifier(cascadePath); font = cv2.FONT_HERSHEY_SIMPLEX #iniciate id counter id = 0 # names related to ids: example ==> Marcelo: id=1, etc names = ['None', 'Wolfgang', 'Bruno', 'Devlyn', 'Z', 'W'] # Initialize and start realtime video capture cam = cv2.VideoCapture(0) cam.set(3, 640) # set video widht cam.set(4, 480) # set video height # Define min window size to be recognized as a face minW = 0.1*cam.get(3) minH = 0.1*cam.get(4) while True: ret, img =cam.read() gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY) faces = faceCascade.detectMultiScale( gray, scaleFactor = 1.2, minNeighbors = 5, minSize = (int(minW), int(minH)), ) for(x,y,w,h) in faces: cv2.rectangle(img, (x,y), (x+w,y+h), (0,255,0), 2) id, confidence = recognizer.predict(gray[y:y+h,x:x+w]) # Check if confidence is less them 100 ==> "0" is perfect match if (confidence < 100): id = names[id] confidence = " {0}%".format(round(100 - confidence)) else: id = "unknown" confidence = " {0}%".format(round(100 - confidence)) cv2.putText(img, str(id), (x+5,y-5), font, 1, (255,255,255), 2) cv2.putText(img, str(confidence), (x+5,y+h-5), font, 1, (255,255,0), 1) cv2.imshow('camera',img) k = cv2.waitKey(10) & 0xff # Press 'ESC' for exiting video if k == 27: break # Do a bit of cleanup print("\n [INFO] Exiting Program and cleanup stuff") cam.release() cv2.destroyAllWindows()
PythonEngine1.ExecStrings(SynEdit1.Lines);
Project Project1.exe raised exception class EPyException with message 'error: OpenCV(4.6.0) D:\a\opencv-python\opencv-python\opencv_contrib\modules\face\src\facerec.cpp:61: error: (-2:Unspecified error) File can't be opened for reading! in function 'cv::face::FaceRecognizer::read'
'. -
Can this part be displayed in a Delphi TImage component ?
cv2.imshow('camera',img)
Is it possible?
-
All of the commercially available solutions are either not available for Delphi or are prohibitively expensive.
Using Python4Delpy is not only accurate, but also cost free. The following Python Script (OpenCV based) is, what I am working on:
The lines towards the bottom:
cv2.imshow('camera',img) stream = BytesIO(img) stream.getvalue()
would be ideal, if I could show the Image in a TImage in Delphi rather than in the Window created by the script
'''' Real Time Face Recogition ==> Each face stored on dataset/ dir, should have a unique numeric integer ID as 1, 2, 3, etc ==> LBPH computed model (trained faces) should be on trainer/ dir Based on original code by Anirban Kar: https://github.com/thecodacus/Face-Recognition Developed by Marcelo Rovai - MJRoBot.org @ 21Feb18 ''' import cv2 import io import numpy as np import os from PIL import Image from io import BytesIO import face_recognition import sys recognizer = cv2.face.LBPHFaceRecognizer_create() recognizer.read('trainer/trainer.yml') cascadePath = "haarcascade_frontalface_default.xml" faceCascade = cv2.CascadeClassifier(cascadePath); font = cv2.FONT_HERSHEY_SIMPLEX #iniciate id counter id = 0 # names related to ids: example ==> Marcelo: id=1, etc names = ['None', 'Wolfgang', 'Bruno', 'Devlyn', 'Z', 'W'] # Initialize and start realtime video capture cam = cv2.VideoCapture(0) cam.set(3, 640) # set video widht cam.set(4, 480) # set video height # Define min window size to be recognized as a face minW = 0.15*cam.get(3) minH = 0.15*cam.get(4) while True: ret, img =cam.read() gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY) faces = faceCascade.detectMultiScale( gray, scaleFactor = 1.2, minNeighbors = 5, minSize = (int(minW), int(minH)), ) for(x,y,w,h) in faces: cv2.rectangle(img, (x,y), (x+w,y+h), (0,255,0), 2) id, confidence = recognizer.predict(gray[y:y+h,x:x+w]) # Check if confidence is less them 100 ==> "0" is perfect match if (confidence < 100): id = names[id] confidence = " {0}%".format(round(100 - confidence)) else: id = "unknown" confidence = " {0}%".format(round(100 - confidence)) cv2.putText(img, str(id), (x+5,y-5), font, 1, (255,255,255), 2) cv2.putText(img, str(confidence), (x+5,y+h-5), font, 1, (255,255,0), 1) cv2.imshow('camera',img) stream = BytesIO(img) stream.getvalue() k = cv2.waitKey(10) & 0xff # Press 'ESC' for exiting video if k == 27: break # Do a bit of cleanup print("\n [INFO] Exiting Program and cleanup stuff") cam.release() cv2.destroyAllWindows()
-
I have created a Python Script which uses a WebCam and beautifully displays the captured frames with cv2.imshow('camera',img).
Is it possible to redirect the image to a Delphi Application's TImage?
WebCam Output
in Python4Delphi
Posted
I need to be able to get the name, date and time of an identified person into a Delphi Application for further processing i.e. Wage Calculation and various reports.
It can run standalone, as long as I can communicate with the App in Real Time.