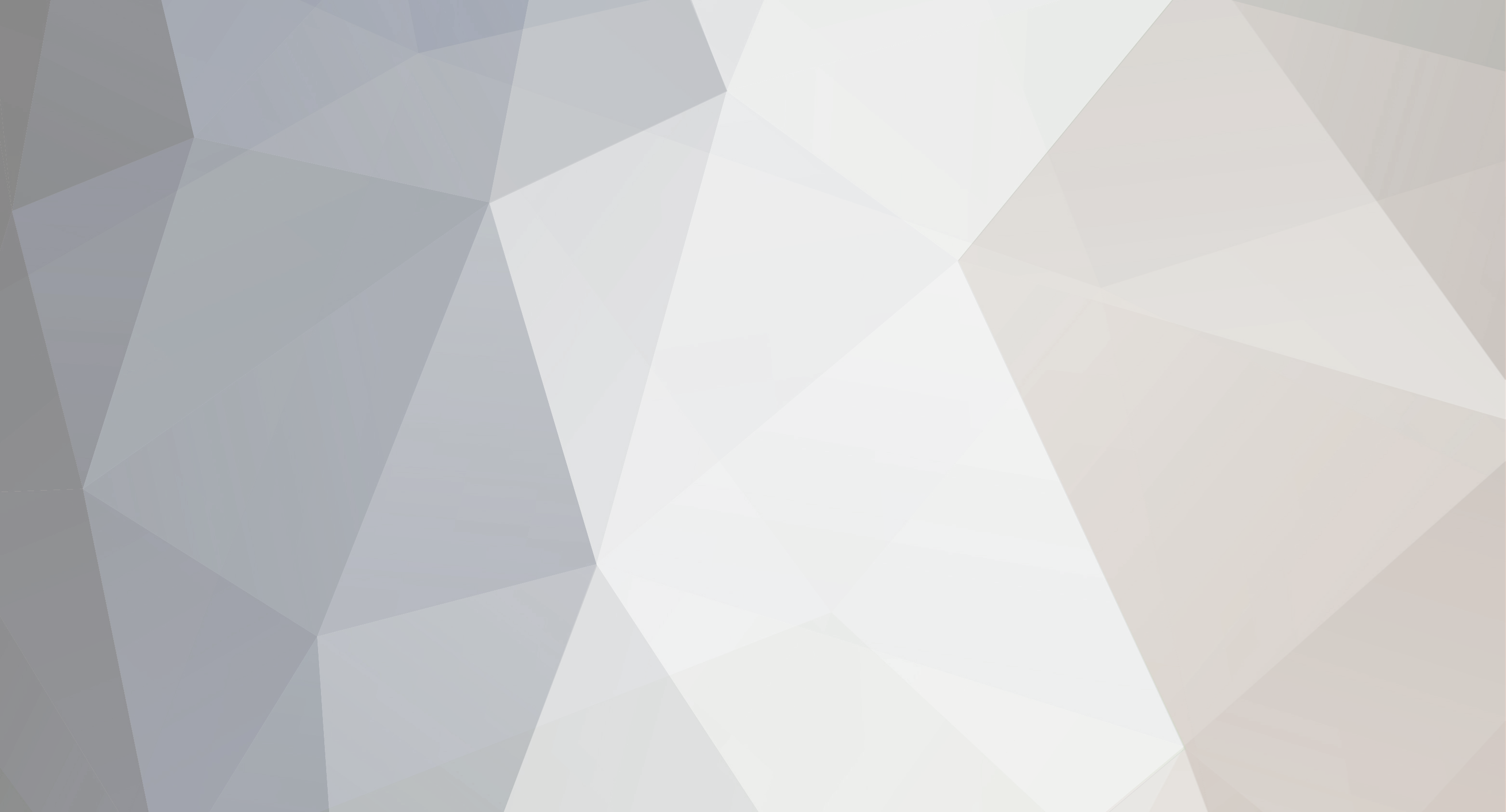
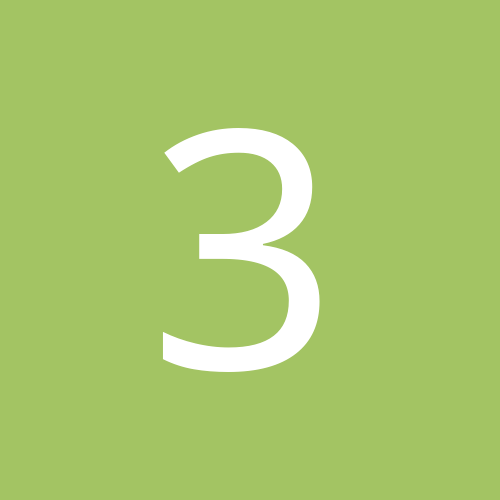
357mag
Members-
Content Count
111 -
Joined
-
Last visited
Community Reputation
3 NeutralRecent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
-
I have been able to successfully step into or trace into but I can't recall how I did it.
-
Same thing happens. If I put the breakpoint next to while(num) and hit F7, the same window with all those crazy addressess shows up. If I put the breakpoint next to the function call square(nums) I get this screen:
-
I put my breakpoint on the function header: void square... ...and when I pressed Trace Into I got all that crazy stuff that looked like addresses.
-
I can't use Trace Into because this is what I see when I use it:
-
Yes num reaches 0. I'm just saying when I put all this stuff on paper, it seems after the last square has been printed, there still is one iteration left to go. So I end up with while(1) instead of while(0). But it's possible that my paperwork was just off a little. I understand how the program works.
-
Plus I was trying to use the debugger so I could watch the value of num in the while loop but there is no step into command. Just step over and trace into. Actually, I've never used a debugger, so I'm new to that. I'm pretty close on this maybe my paperwork is just a bit off.
-
It seems I'm always off by one. This program changes the contents of an array using a function and a pointer. The problem is the while loop in the function. At some point the value of num has to become 0 for the loop to fail and stop printing numbers. But on paper when I plot this thing out, I have already printed all 10 squares 1 through 10, but the value in num is still 1. Shouldn't at that point the value of num be 0? But I keep getting 1. So it's like all 10 squares have been printed but the condition in my while loop looks like this: while(1) instead of... while(0) Here is the code. Sorry I have not changed the namespace stuff yet: void square(int *n, int num); int main() { int i, nums[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; cout << "Original array values: "; for(i = 0; i < 10; i++) cout << nums[i] << " "; cout << endl; square(nums, 10); cout << "Altered array values: "; for(i = 0; i < 10; i++) cout << nums[i] << " "; cout << endl << endl; system("pause"); return 0; } void square(int *n, int num) { while(num) { *n = *n * *n; num--; n++; } }
-
Cool program which I like using a global variable. But the compiler says reference to count is ambiguous. It is one of Herb's programs, and I know his style of writing program is suspect, but his programs themselves as far as his ideas I have always liked. Here it is: #include<iostream> using namespace std; void functionOne(); void functionTwo(); int count; int main() { int i; for(i = 0; i < 10; i++) { count = i * 2; // reference to count is ambiguous functionOne(); } system("pause"); return 0; } void functionOne() { cout << "count: " << count; cout << endl; functionTwo(); } void functionTwo() { int count; for(count = 0; count < 3; count++) cout << '.'; }
-
Excuse me sir for asking a question. Jump in a lake.
-
I'm trying to understand some basic pointer arithmetic. I guess when a pointer is incremented (like ptr++) it does not get incremented by 1. Instead the address increases by 1 multiplied by the size of the data type it is pointing to. If ptr initially held an address called 2000, then ptr++ would make it point to address 2004. What I don't understand is I read "each time ptr gets incremented, it will point to the next integer." What does that mean? The next integer? What next integer?
-
Okay I got it going now.
-
I want to print a line of text using cout but there is a word that I want to have quotation marks around it. I want the output to look like this: Looking for the index position of the word "own". What I've tried so far has not worked. Here is what I got currently: int main() { string sentence = "To thine own self be true"; string word = "own"; cout << "The sentence is: " << sentence << endl; cout << "Looking for the index position of the word \"own\"; cout << sentence.find(word) << endl; system("pause"); return 0; }
-
How do I use a range-based for loop to sum all elements
357mag replied to 357mag's topic in General Help
Yes that works great. Thank You. -
Just one question about the way this program is working. I make a constant and initialize it to 10. I use that to make the size of the array called name1. What I don't understand though is if I run the program and enter in a long name that is longer than 10 characters, C++ still allows me to do it and the program works just fine. I was expecting an error message saying something about 'out of bounds error". I don't get why C++ is allowing me to enter in a name that is longer than the size of the array: #include<iostream> #include<cstring> using namespace std; int main() { const int SIZE = 10; char name1[SIZE]; char name2[SIZE] = "C++owboy"; cout << "Howdy! I'm " << name2 << "!"; cout << " What's your name?" << endl; cin >> name1; cout << "Well, " << name1 << " your name has "; cout << strlen(name1) << " letters" << endl; cout << "Your initial is " << name1[0] << endl; name2[3] = '\0'; cout << "Here are the first three characters of my name: "; cout << name2 << endl << endl; system("pause"); return 0;
-
How do I use a range-based for loop to sum all elements
357mag replied to 357mag's topic in General Help
Something is still off. My console window is saying that sum is equal to 1985788.