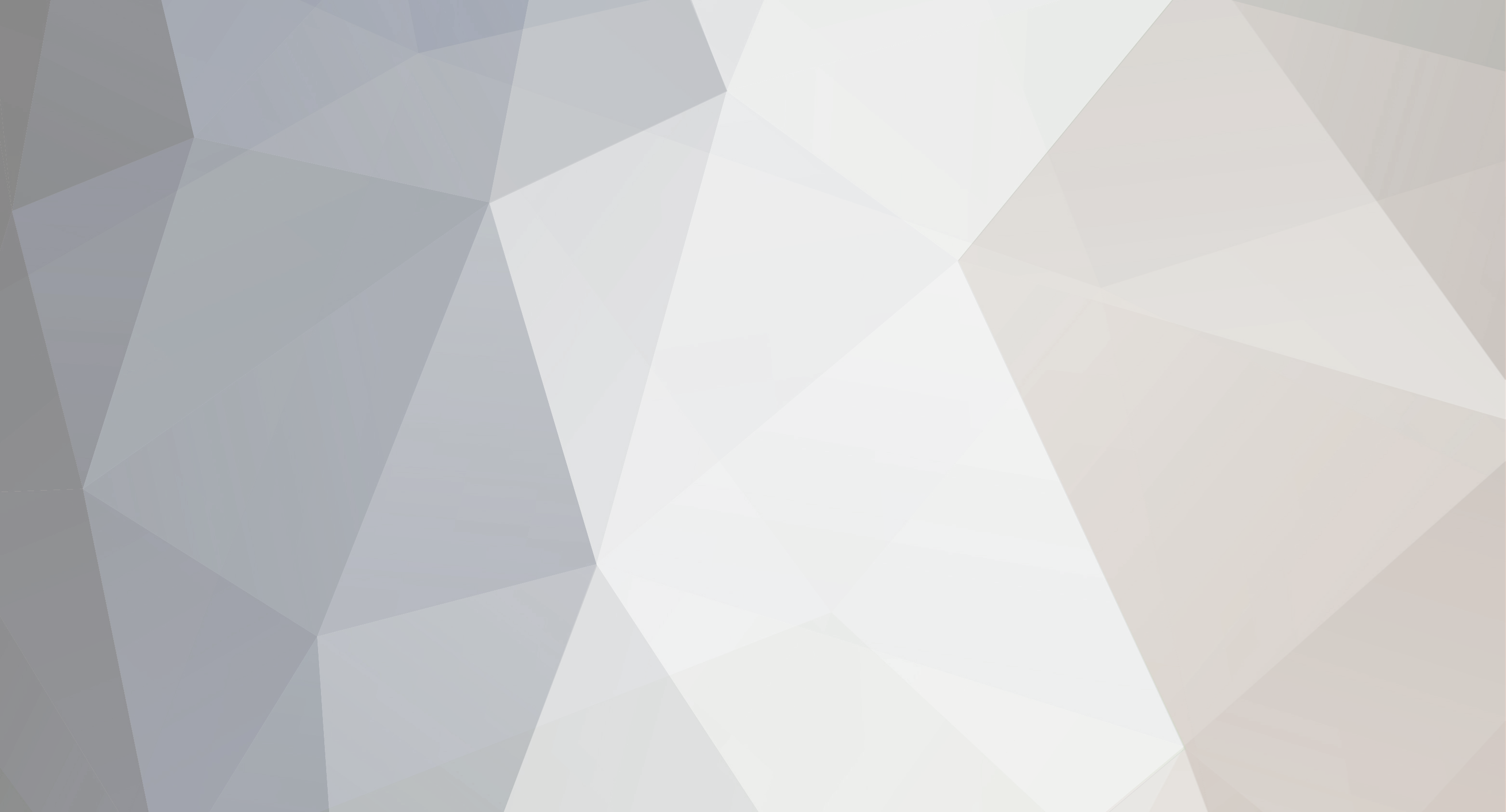
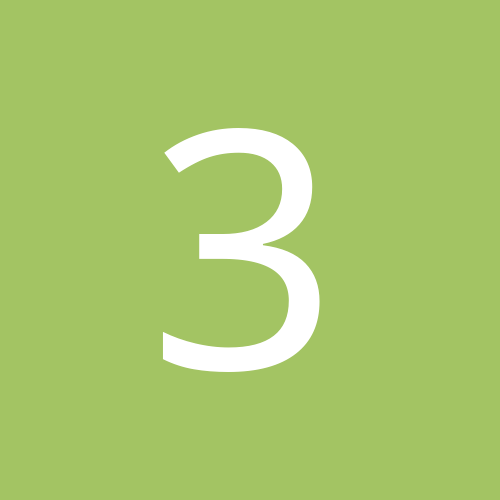
357mag
Members-
Content Count
111 -
Joined
-
Last visited
Everything posted by 357mag
-
The Visual in Visual Basic or Visual C++ or Visual C# simply means you can make a Graphical Interface with it. You're working in a visual manner instead of in a console manner (although you can do that too).
-
No Visual C++ as it comes in Visual Studio and when it was available as a separate product in a box called Visual C++ 6. It was MFC which looked very difficult to monkey with or learn. Now we have WPF and WinForms so maybe it's not so bad. But I don't think Microsoft really wants programmers to use it. They are pushing Visual C#.
-
I need to convert a number (which I think defaults to a string) to an integer in C++ Builder: void __fastcall TForm1::Button1Click(TObject *Sender) { int x; int y; int sum; x = std::stoi (Edit1->Text); y = Edit2->Text; sum = x = y; Label3->Caption = "The sum is" + sum;
-
In order not to piss anyone off on the forum, I will change my code to the C++ Builder string type.
-
I've got a program where the user enters his first name in an Edit box. Then he presses the button entitled Show Message and I will show him a Welcome Message. I'm having some trouble with coding the first name. Builder says Could not find a match for string::basic(const string&). Here is what I have so far: #include <vcl.h> #pragma hdrstop #include "welcome_program.h" #include <iostream> #include <string> using namespace std; //--------------------------------------------------------------------------- #pragma package(smart_init) #pragma resource "*.dfm" TFormWelcome *FormWelcome; //--------------------------------------------------------------------------- __fastcall TFormWelcome::TFormWelcome(TComponent* Owner) : TForm(Owner) { } //--------------------------------------------------------------------------- void __fastcall TFormWelcome::ButtonShowMessageClick(TObject *Sender) { string firstName = AnsiString(EditFirstName->Text); }
-
I got it going! This works: #include <vcl.h> #pragma hdrstop #include "welcome_program.h" #include <iostream> //--------------------------------------------------------------------------- #pragma package(smart_init) #pragma resource "*.dfm" TFormWelcome *FormWelcome; //--------------------------------------------------------------------------- __fastcall TFormWelcome::TFormWelcome(TComponent* Owner) : TForm(Owner) { } //--------------------------------------------------------------------------- void __fastcall TFormWelcome::ButtonShowMessageClick(TObject *Sender) { AnsiString firstName = EditFirstName->Text; LabelMessage->Caption = "Welcome to C++ Programming " + firstName + "!"; }
-
I added Ansistring(sum) and it worked.
-
Well I think I got part of it going. y = Edit2->Text.ToInt(); I think that is okay, but the answer in the Label just says "is". I think I got the same problem going on. Pointer arithmetic or something. I'll have to mess with it.
-
I've got a simple Welcome Message program and when I click the Show Message button it prints a "Welcome to C++ Builder!" message in a label. Only thing that's not going quite right is I would like the text of the message to print in blue, not black. I've got the label selected and I'm going into the color property and choosing clNavy. But when I run the program the Welcome message still prints in black.
-
It works! Thank You So Much.
-
I've wondered if I should do this: EditPrompt->Text or this by adding some whitespace: EditPrompt -> Text How do you guys do it or is there a convention?
-
I can't post the code at the moment, but I wrote a program that inputs a number and then it determines if the number is odd or even. I used a if-else structure. At the end of the code I put and end(with a semi-colon), and immediately after that I put an end(with a period). The compiler complained. It said "expected a . but got a ; instead. I thought you were supposed to use begin and end(with a semi-colon) kind of like an opening brace and a closing brace in other languages. But I had to completely remove the end(with the semi-colon) to get rid of that error. I don't understand why. And then I had a semi-colon on the statement right before the else. Then I find out by reading that you don't do that either. No semi-colon there.
-
This code is the corrected version: var x: integer; begin Write('Enter an integer: '); ReadLn(x); if (x mod 2 = 0) then WriteLn('The number is even') else WriteLn('The number is odd'); WriteLn; Write('Press Enter to quit...'); ReadLn; end.
-
This code is the incorrect version. This is the version I first came up with: var x: integer; begin Write('Enter an integer: '); ReadLn(x); if (x mod 2 = 0) then WriteLn('The number is even'); else WriteLn('The number is odd'); WriteLn; Write('Press Enter to quit...'); ReadLn; end; end.
-
What are some of the ways I can code my console program to keep the console window open after it opens? I know in C++ I used to use getch().
-
I've got a program that asks the user to enter a value (in an Edit box) and then when he clicks on the Show Value button, the Label should say "The value of x is " plus whatever value he entered in the Edit box. But Delphi is complaining saying "x is not a valid integer value." I don't know what's wrong with my code but here is what I've got: var FormSimpleVariable: TFormSimpleVariable; x : integer; implementation {$R *.dfm} procedure TFormSimpleVariable.ButtonQuitClick(Sender: TObject); begin Application.Terminate; end; procedure TFormSimpleVariable.ButtonShowValueClick(Sender: TObject); begin x := StrToInt('x'); LabelResult.Caption := 'The value of x is ' + IntToStr(x); end; end.
-
So when your program is all completed as far as writing all the code, you put an end (with a period) at the end. If you are just denoting a code block, then you put and end (with a semicolon).
-
Yes I was about to post that I found a way using ReadLine. I'm a little confused about the two end keywords. It seems sometimes I see end with a semicolon and that is all. Other times I see end with a period. Other times I see both in a code snippet.
-
I don't recall adding those single quotes around x (like ('x')). Don't know what happened there. Mistakes and being human I guess.
-
My program prompts the user to enter a character (like 'A' for example). Then I'm trying to store it in a character variable. But C++ is telling me it can't cast from Unicode string to a character. How do I handle it? My code looks like this: char ch = 0; int chValue = 0; LabelPrompt -> Caption = "Enter a character: "; ch = static_cast<char>(EditCharacterEntry -> Text); The goal of this program is to print the Ascii code for the letter the user entered. Then it would show something like 'w' is 119. 'x' is 120.
-
Your first code example does not work. C++ Builder wrote an extremely long line complaining about something. It also said chValue is assigned a value that is never used. Your second code example worked though.
-
You guys are acting like jerks. I'm talking about a different piece of code now. If you can't or don't know how to be nice and respectful, then please go to bed. In C++ you can assign a value to a variable immediately after declaring it. Delphi would not let me do that. I had to assign the values to the variables further down underneath the begin keyword.
-
You guys are acting like jerks. I'm talking about a different piece of code now. If you can't or don't know how to be nice and respectful, then please go to bed.
-
How come you can't assign a value to a variable in the same section you declared it? You gotta assign the value underneath the begin keyword instead.
-
So I'm just getting started with Delphi and I went into Options -> Environment Options and under the Default Project list box I told it to save my files to D:\Delphi Programming Projects. In that folder I have two subfolders, one called Console Projects and the other called Windows Projects. I wrote a project today and when I was done I hit Save Project As... and it prompted me to give the unit file a name which I did and a project file a name which I did. And I directed Delphi to the exact folder (which is called Welcome Program) to save the files in. Problem is Delphi also save files and a Win32 folder in the Delphi Programming Projects folder. I need to save everything to the Welcome Program folder, so I don't have files scattered in the Delphi Programming Projects plus the Welcome Program folder too. I can understand why it did that because after all I told Delphi in Environment Options to use the D:\Delphi Programming Projects folder as a default directory. But isn't there a way to get Delphi to put everything in a subfolder? Like in my case the name of my program is Welcome Program. I want everything going into that subfolder. I've enclosed a screenshot.