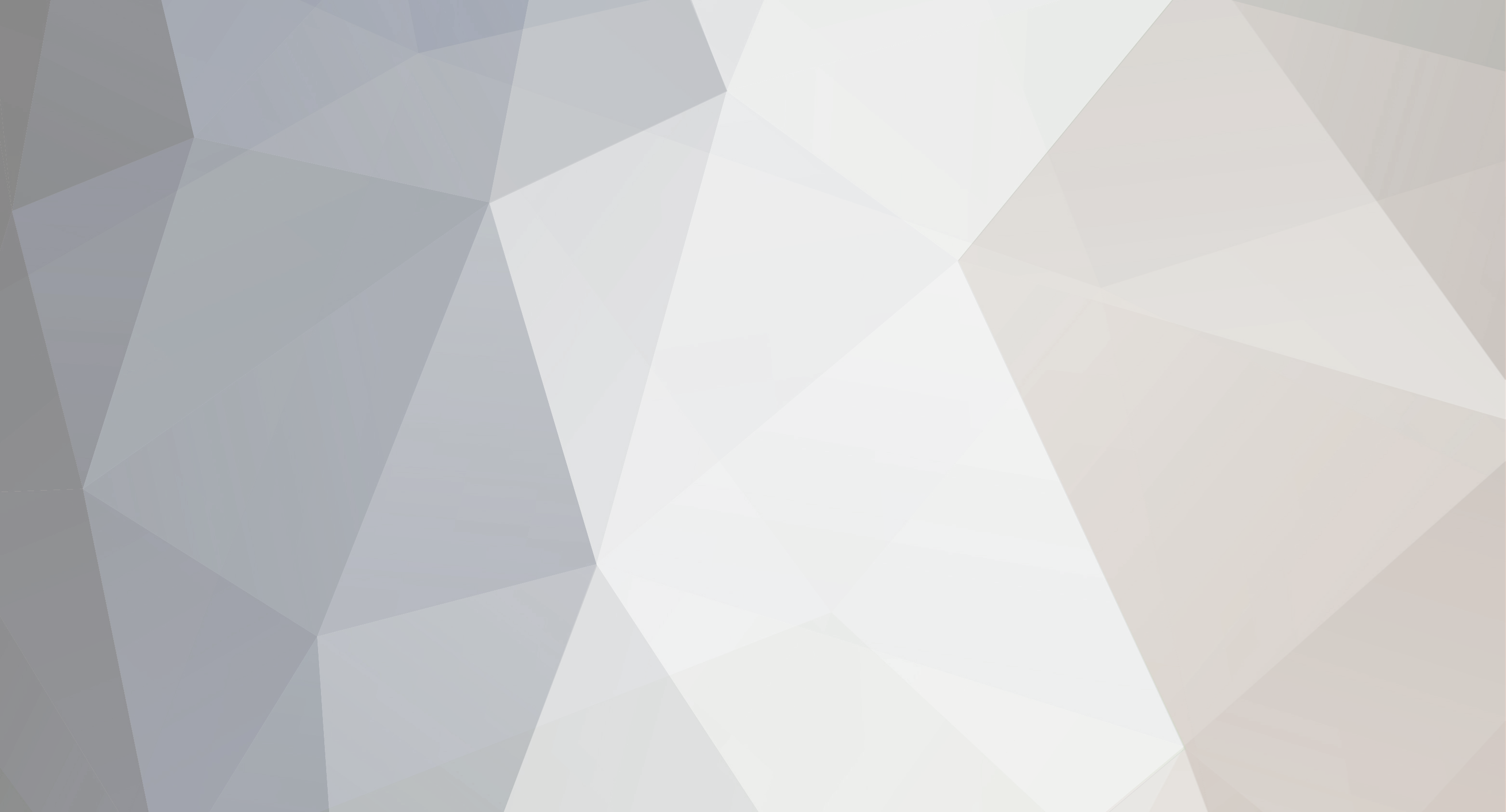
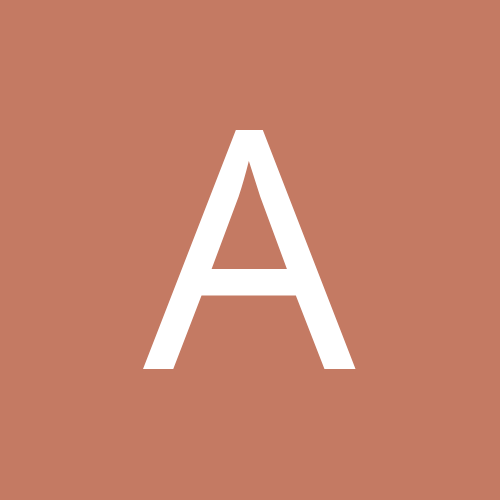
AaronCatolico1
Members-
Content Count
4 -
Joined
-
Last visited
Everything posted by AaronCatolico1
-
*UPDATED! So, the problem is that this particular listview is different than the VCL Listview. I guess FMX does things differently when populating this listivew. Does anyone know how to add the subitems or is it just not an option due to mobile applications? How do I add in the columns along with the rows? I'm new to using Delphi and RAD Studio, so this has been a little bit of a learning curve for me, especially when most sources online say to write the syntax in a particular way and it only throws errors: Anyhow, I was able to populate the ListView with only a single column and multiple rows. Most sources online say that this is how you populate a listview in a buttonclick event: procedure TForm1.Button1Click(Sender: TObject); var Item: TListViewItem; {Or possibly use the following:} Item: TListItem; begin Item := ListView1.Items.Add; item.text := 'dude'; item.subitems.add('bro'); end; end. Every time that I try to use the 'item.subitems.add', it throws the error: 'Undeclared Identifier' : 'subitems'. Can someone show me an example of how to populate a listview control with items and subitems? Just a very simple example is all I need. A good example would be 4 columns and 4 rows of data. Thanks. *I'm using RAD Studio 12 with firemonkey.
-
I'm new to using Delphi with RAD Studio. I would like someone to show me a basic example of how to use a thread. I've been looking for examples online for a very basic example and haven't found anything good. I want to have a simple Label1 and a Button1 on a form. When I click the button, I'd like to see Label1 increment up by 500 milliseconds on it's own thread. Can someone show me a very basic example. I'd greatly appreciate it. Here's what I've tried, but keep getting the error message: "E2036: variable needed" right next to the Synchronize($UpdateUI) unit Unit1; interface uses System.SysUtils, System.Types, System.UITypes, System.Classes, System.Variants, FMX.Types, FMX.Controls, FMX.Forms, FMX.Graphics, FMX.Dialogs, FMX.Controls.Presentation, FMX.StdCtrls, System.SyncObjs; type TForm1 = class(TForm) Button1: TButton; Label1: TLabel; procedure Button1Click(Sender: TObject); private { Private declarations } Counter: Integer; public { Public declarations } end; { TMyThread is the class that will handle our background work } MyThread = class(TThread) private x: Integer; msg: string; protected procedure Execute; override; procedure UpdateUI; public constructor Create; end; var Form1: TForm1; implementation {$R *.fmx} {Create the TThread} constructor MyThread.Create; begin inherited Create(False); // False means it will start right away FreeOnTerminate := True; // Free memory when finished end; {Worker Thread} procedure MyThread.Execute; var i:integer; begin // Simulate some work by looping and sleeping for i := 1 to 1000 do begin Sleep(10); // Simulate work (1 second per iteration) msg := '# ' + IntToStr(i); Synchronize(@UpdateUI); // Update UI safely from the main thread end; msg := 'FINISHED!'; showmessage('FINISHED!!!'); Synchronize(@UpdateUI); end; {Update the UI} procedure MyThread.UpdateUI; begin Form1.Label1.Text := inttostr(x); end; procedure TForm1.Button1Click(Sender: TObject); begin MyThread.Create; // Create and start the thread end; end.
-
Thank you! I discovered this to be the case the other day.
-
I have a button1 and a label1. When the button1 is clicked, the label1 starts counting up on it's own thread. The issue is that I have NOT been able to stop the thread when button2 is clicked. Here's the code I'm working with: unit Unit1; interface uses System.SysUtils, System.Types, System.UITypes, System.Classes, System.Variants, FMX.Types, FMX.Controls, FMX.Forms, FMX.Graphics, FMX.Dialogs, FMX.Controls.Presentation, FMX.StdCtrls, System.SyncObjs; type TForm1 = class(TForm) Button1: TButton; Label1: TLabel; Button2: TButton; procedure Button1Click(Sender: TObject); procedure Button2Click(Sender: TObject); private public end; { TMyThread is the class that will handle background work for Label1 } MyThread1 = class(TThread) private count: Integer; msg: string; stopThread:boolean; protected procedure Execute; override; procedure UpdateUI; public constructor Create; end; var Form1: TForm1; implementation {$R *.fmx} { MyThread Constructor } constructor MyThread1.Create; begin inherited Create(False); // False means it will start right away FreeOnTerminate := True; // Free memory when finished end; { Worker Thread for Label1 } procedure MyThread1.Execute; var i: Integer; begin for i := 1 to 1000 do begin //Check to see if the was terminated. If so, then exit the for loop. if Terminated then Exit; Sleep(10); // Simulate work (1 second per iteration) msg := '# ' + IntToStr(i); Synchronize(UpdateUI); // Update UI safely from the main thread end; Synchronize(UpdateUI); end; { Update the UI for Label1 } procedure MyThread1.UpdateUI; begin Inc(count); Form1.Label1.Text := msg; //WHen finished: if (count = 1000) then begin ShowMessage('Thread 1 Finished!'); end; end; {Start the thread when button1 is clicked} procedure TForm1.Button1Click(Sender: TObject); begin MyThread1.Create; // Create and start the first thread for Label1 end; procedure TForm1.Button2Click(Sender: TObject); //Procedure to stop the MyThread1 thread goes here. begin end; end.