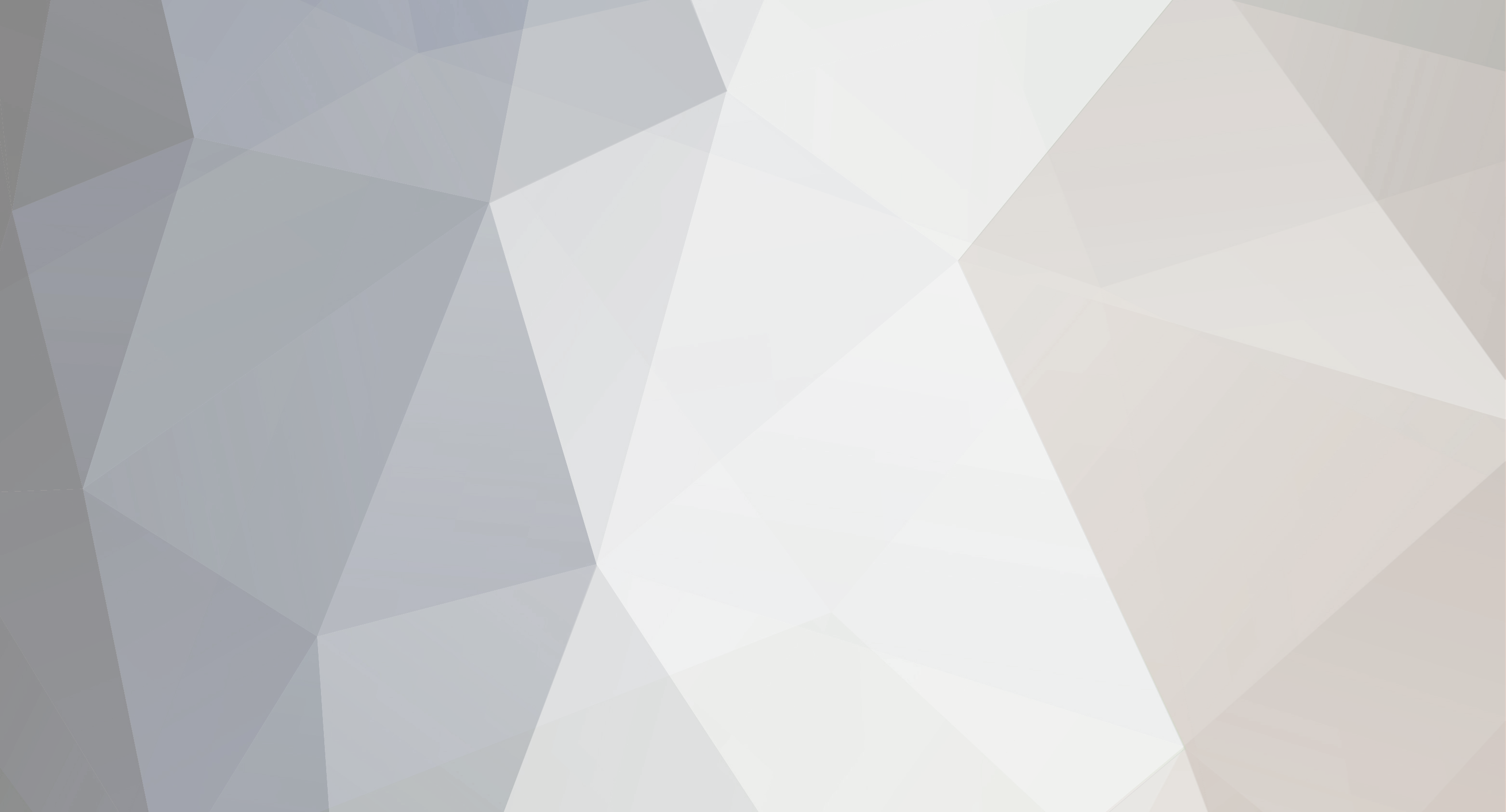
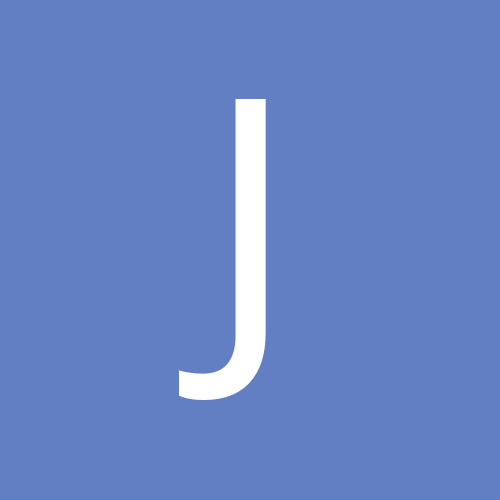
John Gambler
Members-
Content Count
4 -
Joined
-
Last visited
Everything posted by John Gambler
-
I'm having some difficulty using variables through their memory addresses. In the test below, the pointer of the ParamEmbeddedRec variable and the Params variable (inside ParamMaster) should be the same, so the value of PatchParam and Payload (inside ParamEmbeddedRec) are invalid. I think the syntax of line 47 (ParamMaster.Params := @ParamEmbedded) is not correct. Bellow, my code and an image of the debug, My code: unit Unit1; interface uses Winapi.Windows, Winapi.Messages, System.SysUtils, System.Variants, System.Classes, Vcl.Graphics, Vcl.Controls, Vcl.Forms, Vcl.Dialogs, Vcl.StdCtrls; type TForm1 = class(TForm) Button1: TButton; procedure Button1Click(Sender: TObject); private { Private declarations } public { Public declarations } end; var Form1: TForm1; implementation {$R *.dfm} procedure TForm1.Button1Click(Sender: TObject); Type TParamMaster = Record Operacao : Smallint; Params : Pointer; End; Var ParamMaster : ^TParamMaster; Type TParamEmbedded = Record PatchParam : String; Payload : String; End; Var ParamEmbedded : ^TParamEmbedded; Var ParamEmbeddedRec : ^TParamEmbedded; begin New(ParamMaster); New(ParamEmbedded); ParamEmbedded.PatchParam := 'somePatch'; ParamEmbedded.Payload := '{"aooCode": "F60EB930-9B1B-11EF-9657-02240D86274B"}'; ParamMaster.Operacao := 1; ParamMaster.Params := @ParamEmbedded; ParamEmbeddedRec := ParamMaster.Params; Dispose(ParamEmbedded); Dispose(ParamMaster); end; end.
-
Perfect. I'm glad I didn't find this one in my attempts, otherwise I wouldn't have had access to such a comprehensive explanation. I use this approach to access a DLL from my application. This DLL only exports one function, which receives a pointer and returns a pointer. ParamMaster represents the generic parameter for the DLL. ParamMaster.Operation represents which service from the DLL I want to use and ParamMaster.Param represents the parameter used by this service. The DLL returns a pointer that represents the response from this service. Right after calling the service, the caller copies the response to internal variables and calls the DLL again to inform it that it no longer needs the response variable and the DLL calls a dispose for it. Right after, the caller disposes the parameter variable. I don't know if I was clear. In any case, I'm satisfied with the quick and objective response. Thank you.
-
I have a small program(code bellow) to communicate with a rest API, everything works fine. My problem now is how can i fix the response values with diacritic character. Examples: correct string: não - received string: n\u00E3o correct string: padrão - received string: padr\u00E3o My code: unit Unit1; interface uses System.SysUtils, System.Types, System.UITypes, System.Classes, System.Variants, FMX.Types, FMX.Controls, FMX.Forms, FMX.Graphics, FMX.Dialogs, FMX.Edit, FMX.Controls.Presentation, FMX.StdCtrls, REST.Types, Data.Bind.Components, Data.Bind.ObjectScope, REST.Client, FMX.Memo.Types, FMX.ScrollBox, FMX.Memo, System.JSON; type TForm1 = class(TForm) Button1: TButton; Button2: TButton; Memo1: TMemo; procedure Button1Click(Sender: TObject); procedure Button2Click(Sender: TObject); procedure Send(Url : String); private { Private declarations } public { Public declarations } end; var Form1: TForm1; implementation {$R *.fmx} procedure TForm1.Send(Url : String); Var myClient : TRESTClient; myRequest : TRESTRequest; myResponse : TRESTResponse; Content : String; Begin myRequest := TRESTRequest.Create(Application); myResponse := TRESTResponse.Create(Application); myClient := TRESTClient.Create(nil); myRequest.Client := myClient; myRequest.Response := myResponse; Try myRequest.AddParameter('Content-Type', 'application/json', TRESTRequestParameterKind.pkHTTPHEADER, [poDoNotEncode]); myRequest.AddParameter('Accept', 'application/json', TRESTRequestParameterKind.pkHTTPHEADER, [poDoNotEncode]); myRequest.Method := TRESTRequestMethod.rmPOST; Content := '{"emptyString": "", "someUuid": "F60EB930-9B1B-11EF-9657-02240D86274B"}'; myRequest.Params.AddItem('body',Content,TRESTRequestParameterKind.pkREQUESTBODY,[],ctAPPLICATION_JSON); myRequest.Resource := Url; myRequest.Execute; Memo1.Lines.Add('objectProperty1: ' + (myResponse.JSONValue as TJSONObject).GetValue('objectProperty1').ToJSON()); Memo1.Lines.Add('objectProperty2: ' + (myResponse.JSONValue as TJSONObject).GetValue('objectProperty2').ToJSON()); Finally Resposta.Free; myRequest.Free; myClient.Free; End; End; procedure TForm1.Button1Click(Sender: TObject); begin Send('http://localhost:4000/local/'); end; procedure TForm1.Button2Click(Sender: TObject); begin Send('https://mystackurl.com'); end; End.
-
Thank you Remy and Uwe, both ways worked ok.