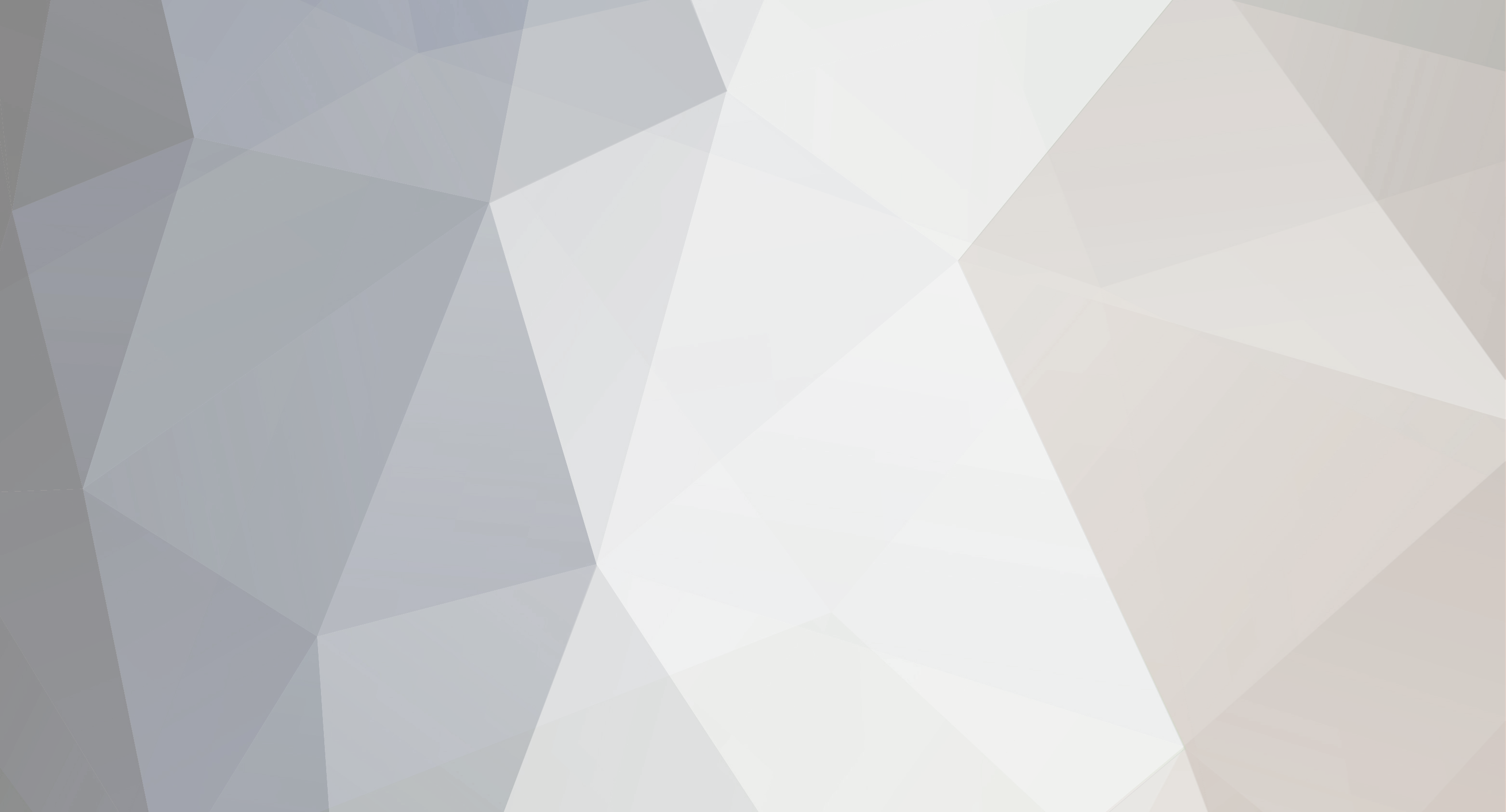
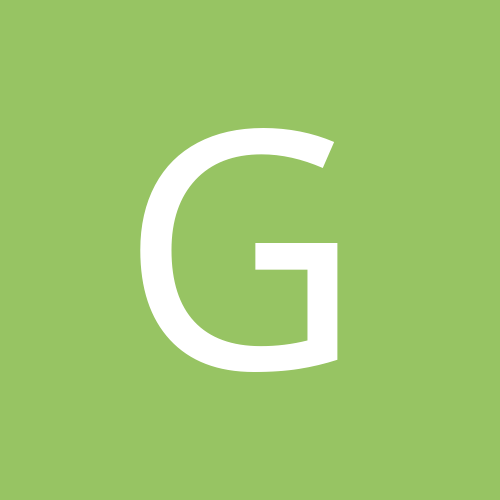
gioma
-
Content Count
143 -
Joined
-
Last visited
-
Days Won
1
Posts posted by gioma
-
-
I tried on "C:\" and it works.
I tried on "C:\Users\MyUser\OneDrive\Desktop" and it works.
i created and tried on "C:\TEST_FT" and it works.It doesn't work where the software was started with a different manifest!
-
I tried, it has no effect.
I opened the files and there doesn't seem to be anything that leads back to those menus, sigh! -
sorry, I was wrong to answer.
-
I just emptied the folder, kept only "Layout.ini" and "PfPre_a24c160c.mkd" but the behavior doesn't change.
In that folder the program generated by Delphi doesn't seem to have the right manifest and therefore if I change the resolution it gets deformed. -
Hi
I changed the screen resolution and the Delphi 11.2 menu got "messed up".I tried to restart Delphi but nothing, now I think those positions and sizes are marked in some configuration file and there's no way to fix it dall'IDE.
Now the only way to work with it is to deactivate the various menusHow can I reset the menus positions/sizes ?
-
Hello everyone,
I have an application developed in Delphi 11.2, I changed the DPI Awareness by selecting the "MonitorV2" option, but the change has no effect on the exe generated in that specific folder.
If instead I move in othrer folder the generated file then everything works fine.
I believe it's the windows cache (in this case windows 11) not allowing to reload the manifest of the file.
I tried restarting the OS, but that didn't work.
Is there a way to solve this problem? -
I found SpyPrinter for Delphis on the embarcadero site that is right for me, if someone else will need it here is the link:
http://cc.embarcadero.com/item/20307
🤩 DAJE!
-
Sounds like a good start, thanks.
-
Greetings,
I would need that my program to be able to detect that a print has been launched in the operating system and to which printer.
I was thinking of using HOOKs, like I do for keyboard and mouse events.
I was studying the hooks available on Windows but I still haven't been able to figure out which one can do for me.Can any of you give me some suggestions? Thank you
-
5 hours ago, PeterBelow said:When you deverlop a custom component it is a good idea to use a test project first in which you create an instance of the component in code. This allows you to debug the run-time behaviour of the component without endangering the IDE itself. Only when everything works as it should do you add it to a design-time package and install it, to validate the design-time behaviour. If you need to add a custom property or class editor these can also be developed and tested in the test project, by just doing what the IDE designer does for invoking them. I don't remember the details since it has been literally decades since I last needed to do this, but it can be done.
It's a great idea, I did a similar thing, but the IDE got stuck anyway.
-
On 7/15/2022 at 3:16 PM, Anders Melander said:Not very shocking if you understood what was going on. When you install a component your code will be run as a part of the IDE process. If your component code goes into an endless loop then you will have hung the IDE. Nothing surprising there.
So is Delphi. I'm assuming you're a hobbyist because otherwise VS isn't free AFAIK.
I was a hobbyist when I was 12 and I was programming in Basic with the Vic20..
Visual studio professional has a lower price base.
Yes, I understand that the error was caused by me, the IDE must rightly report it to me, but then it cannot go into an infinite loop of errors.
On 7/15/2022 at 3:29 PM, Brian Evans said:I think you would benefit from taking a break and acquiring some Delphi custom component development and debugging skills. Impatience to do something when you have not yet acquired the necessary skills and knowledge usually leads to frustration. The basics of component development and debugging have not changed much so even older books/guides are still useful. Ray Konopka wrote some early books on Delphi component development any which would be a good resource if you can get your hands on one.
I have been developing with Delphi for many years (more than 16), but there are many aspects of programming and you never stop learning.
I don't often have to create a component, I usually buy them, use them and modify them if necessary.
In this case I had to make a component from 0, so I was faced with new problems for the first time.
I am not frustrated, but I expect that if I make a mistake I can analyze it, understand it and fix it.
If the IDE goes into an endless loop of errors I can't do anything anymore.Sometimes for example it happens that when I update the component, if I go to recompile delphi it blocks the BPL file. The only way I have to re-install it is to close and reopen Delphi.
These are time wasters that you would not want to encounter when you have to develop in precise timing.
-
sorry for the outburst, but sometimes it's really frustrating to work with Delphi .. you waste a lot of time with nonsense!
-
The shocking thing though is that if you make a mistake in creating a component and then install it, Delphi goes into an unending error loop and you are forced to close it from the task manager!
The IDE is still not very stable, although it costs a lot and its competitors are free!
I have been using Delphi for 16 years (Delphi 5, 7, delphi 2005 .. up to Delphi 11 Alexandria), in the beginning it was much better than Visual Studio .. now it is far behind and the updates are very, very, very slow to come out .. Visual studio has updates every week!
And I repeat .. Visual Studio is free!
-
19 minutes ago, Anders Melander said:No you don't. I understand why you used it but there are always better ways to solve a problem than using [weak].
Contrary to what the documentation states [weak] isn't named named after the type of reference. It's named after the design skills of people who choose to use it to manage references. 🙂
16 minutes ago, Dalija Prasnikar said:Weak attribute only works on interface references, not on object references. In your case, with TComponent reference, compiler just ignores weak attribute.
Right, you are right!
I have used weak on other occasions with Interfaces, in this case it is a TObject so it makes no sense to use it.
Distraction error
Thanks again! -
On 7/11/2022 at 3:41 PM, Anders Melander said:- Don't insert tabs in your source.
- Don't use [weak]
- Use the notification mechanism built into TComponent:
type TComponentA = class(TComponent, IComponentAl) private FComponentB: TComponentB; private procedure SetComponentB(const Value: TComponentB); protected procedure Notification(AComponent: TComponent; Operation: TOperation); override; published property ComponentB: TComponentB read FComponentB write SetComponentB; end; procedure TComponentA.SetComponentB(const Value: TComponentB); begin if (FComponentB <> nil) then FComponentB.RemoveFreeNotification(Self); FComponentB := Value; if (FComponentB <> nil) then FComponentB.AddFreeNotification(Self); end; procedure TComponentA.Notification(AComponent: TComponent; Operation: TOperation); begin inherited; if (AComponent = FComponentB) and (Operation = opRemove) then FComponentB := nil; end;
I need the weak parameter, because in real use they are two components that refer to each other.
I solved it using the notification mechanism .
Thank you!
-
-
I created two visual components, one referencing the other in the published properties.
Example:
TComponentA = class(TComponent, IComponentAl) [weak] _ComponentB: TComponentB; //...other code private procedure SetCopmponentB ( const Value: TComponentB); //.. other code published property ComponentB: TComponentB read _ComponentB write SetCopmponentB; //.. other code procedure TComponentA.SetCopmponentB(const Value: TComponentB); begin try _ComponentB := Value; //.. other code except on e:exception do WriteLog('[TComponentA.SetCopmponentB] EX: ' + e.Message); end; end;
in the design phase, I add the two components ComponentA: TComponentA and ComponentB: TComponentB in the form.
By Deisngh i set the property ComponentA.ComponentB: = ComponentB.Then if I delete ComponentA from design I have no problems, if instead I delete componentB (which is associated with the property of componentA) then Delphi goes into an exception unended loop.
What should I add to the code to prevent this from happening? -
Eventually I got to the heart of the problem.
There is a thread in the program that uses the pipe to communicate with other programs.
In particular, the program receives a message to its pipe when it is started but an instance of it already exists in that session.
It does the same thing even when it's hidden and double-clicked on the TryIcon.
This message instructs the application to show itself and put itself in the foreground. However, if the program is hidden, this instruction blocks both the pipe thread and the main thread.
There was no synchronization with the main thread. -
Ok, I solved the problem with the example :
procedure TFmainFormDef.FormCreate(Sender: TObject); var T:TThread; begin T:=TThread.CreateAnonymousThread( Procedure begin TThread.Sleep(2000); TThread.Synchronize(nil, procedure begin FmainFormDef.Visible:=true; end); end); T.FreeOnTerminate:=true; T.Start; end;
The statement that makes the form visible had to be synchronized with the main thread.
At this point I think the problem is related to the components. -
in the original project use of graphic components.
In that case I have problems with the visualization of these components (buttons, labels, etc.) ..
All this always if the form has the visible = false property.
It is probably a component problem, but the fact that with standard components I get such an error makes me think there is so much more. -
Simple example:
main Form
unit MainFormDefault; interface uses Winapi.Windows, Winapi.Messages, System.SysUtils, System.Variants, System.Classes, Vcl.Graphics, Vcl.Controls, Vcl.Forms, Vcl.Dialogs, Vcl.Buttons, Vcl.StdCtrls, Vcl.ExtCtrls; type TFmainFormDef = class(TForm) Panel1: TPanel; Panel2: TPanel; ComboBox1: TComboBox; SpeedButton1: TSpeedButton; procedure FormCreate(Sender: TObject); procedure FormClose(Sender: TObject; var Action: TCloseAction); private { Private declarations } public { Public declarations } end; var FmainFormDef: TFmainFormDef; implementation {$R *.dfm} procedure TFmainFormDef.FormClose(Sender: TObject; var Action: TCloseAction); begin Action:=CaFree; end; procedure TFmainFormDef.FormCreate(Sender: TObject); var T:TThread; begin T:=TThread.CreateAnonymousThread( Procedure begin TThread.Sleep(2000); FmainFormDef.Visible:=true; end); T.FreeOnTerminate:=true; T.Start; end; end.
File .dpr
program TestHideMainForm; uses Vcl.Forms, MainFormDefault in 'MainFormDefault.pas' {FmainFormDef}, MainForm in 'MainForm.pas' {FmainForm}; {$R *.res} begin Application.Initialize; Application.MainFormOnTaskbar := True; Application.CreateForm(TFmainFormDef, FmainFormDef); Application.ShowMainForm:=false; FmainFormDef.Visible:=false; Application.Run; end.
Here I start a simple program in hidden mode, then with a simple thread I simulate an event that shows it.
In this case everything goes smoothly but when I close the program I get this error: -
It's a very big project, so it's hard for me post the code.
Basically the program connects to a socket server and performs some operations.
If started by another program, which is installed as a server, the program starts hidden, with an icon on the tray bar.
The operations it performs with the socket server update the interface (with messages, buttons/pannels that become visible / invisible).
If I start the program and the main form has the option visible=true in the object explorer everything works fine.
Otherwise, if the program is hidden, when the interface is shown it presents transparent pieces as if it had not been able to draw it and then freezes until it crashes. -
1 hour ago, Uwe Raabe said:Could it be that you have PrimaryForm.Visible = True in the Object Inspector?
Yes, indeed it is true.
Trivially the problem is that.
But now I have another problem.
I create and modify objects of PrimaryForm at runtime (including frames) and it happens that if I set PrimaryForm.visible = false in the Object Inspector when I make the window visible again (show or visible=true) the program remains blocked, as if it could not redraw the interface.
In the code I have no errors, all the instructions are executed without problems, but when I view the window it remains frozen, as if it could not redraw it. -
Hello everyone,
I'm facing a problem with Delphi Alexandria.
In some cases I would like not to show the main form of the project.
To do this, simply use the following code://... other preliminary operations Application.Initialize; Application.Title := GF_GetAppName; Application.MainFormOnTaskbar := True; Application.CreateForm(TPrimaryForm, PrimaryForm); if pos('-NOSHOW', UpperCase(CmdLine)) > 0 then begin Application.ShowMainForm := False; PrimaryForm.visible:=false; end; Application.Run;
Obviously the project is quite complex, but these instructions should be enough to stop the Main Form from doing the "Show" event.
In debugging, on the other hand, I realized that when it executes the line
Application.CreateForm(TPrimaryForm, PrimaryForm);
The show event of the form is executed, before:
Application.Run;
Then the instructions are executed:
Application.ShowMainForm: = False;
PrimaryForm.visible: = false;which hide the main form, but I didn't want it to be shown at all.
finally executes
Application.Run;
and at this point I expect that the form (if the -NOSHOW parameter is not passed) activates the onShow event and not that it is activated instead by the "Application.CreateForm" statement
Why does this happen? What am I missing?
[DELPHI 11] : menu messed up when changing resolutions/DPI of monitor
in Delphi IDE and APIs
Posted
I clicked on the reset button.. here's the result, the problem I don't think is related to the profile, but he changed a generic menu setting and now there's no way to get him to reset that!