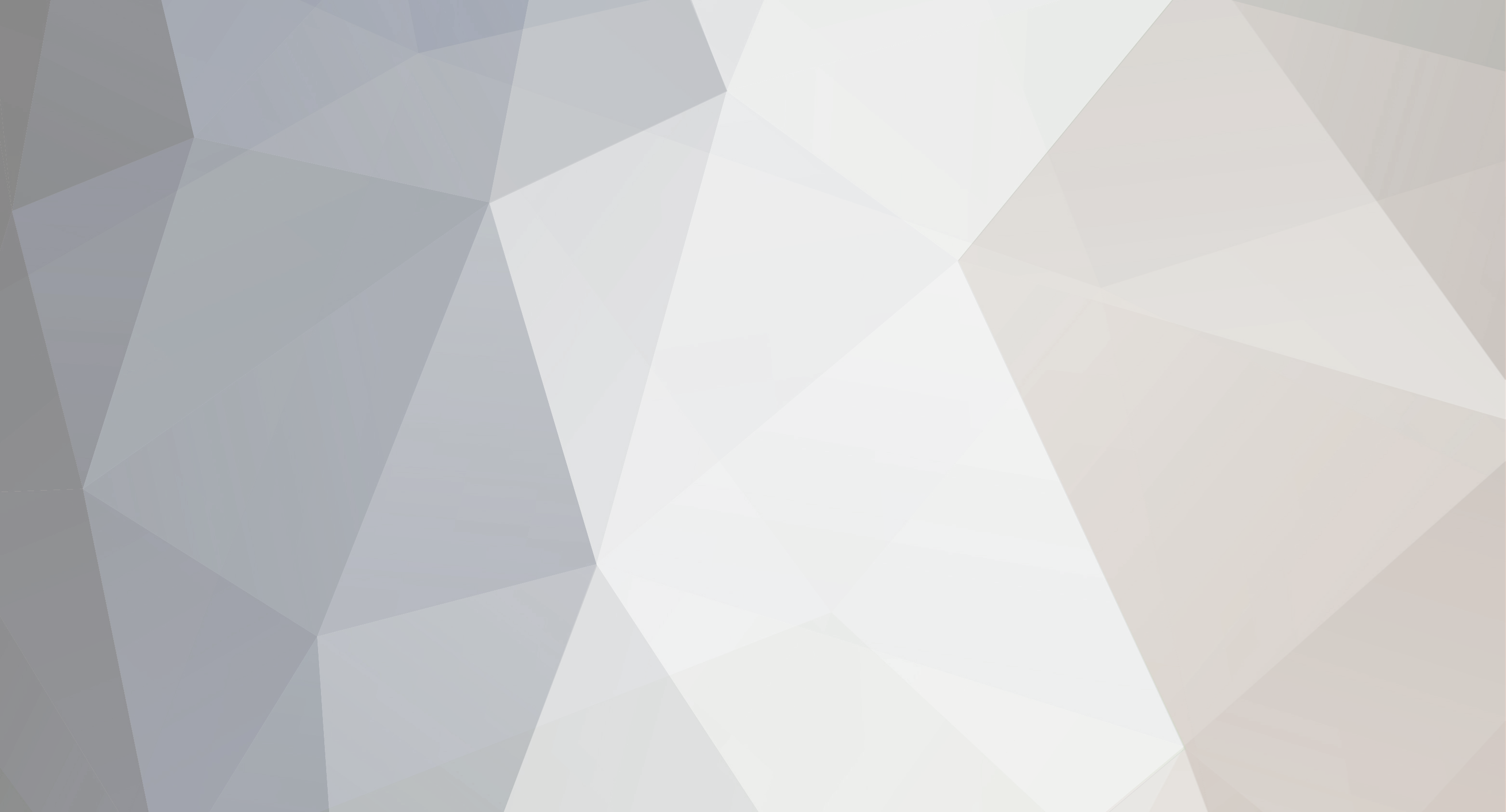
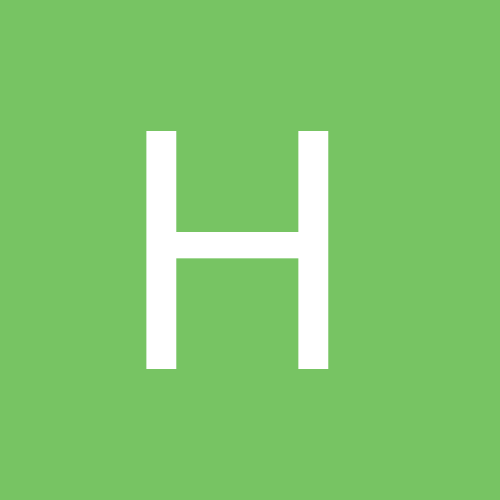
HolgerX
-
Content Count
18 -
Joined
-
Last visited
Posts posted by HolgerX
-
-
Sorry to read this ..
Especially old projects, which cannot be upgraded to a new Delphi version, will probably have to stop at INDY 10 .. -
hmm
I played with TightVNC as a test.
Get the window handle (FindwindowW (..)) from the VNC and then used the following:var VNCHandle : HWND; .. VNCHandle := FindwindowW(nil, PWideChar(WindowCaption)); if VNCHandle <> 0 then begin Windows.SetParent(VNCHandle,Panel1.Handle); WPM.Length:=SizeOf(WPM); GetWindowPlacement(VNCHandle,@WPM); Rect.Top :=0; Rect.Left :=0; Rect.Right :=Panel1.Width; Rect.Bottom:=Panel1.Height; wpm.rcNormalPosition:=Rect; SetWindowPlacement(VNCHandle,@WPM); end;
This shows the VNC window in the panel.
Do this several times, each with its own panel and you can then manipulate the panels with the VNC windows as you like.-
1
-
-
Hmm..
Or if you only need TLS 1.2 INDY should an option.
With the OpenSSL Libs Up to TLS 1.2 could be used insteed of the libs from the OS.
This should than run with WinXP.
-
Hmm..
Unfortunately, this was not possible with a simple script.
I had a simple tool written, which after defrag (with move to the beginning!) Just new files (each 40 MB size) with always # 0 as a character has generated.
Thus I filled the 'empty' area of the hard disk completely with # 0. Finally, just delete these files again.
Was just a workarround for VirtualPC ..
With the DiskManager of VirtualPC, the physical size of the hard disk could be reduced.
Do you already know this link?
https://virtualman.wordpress.com/2016/02/24/shrink-a-vmware-virtual-machine-disk-vmdk/
This was linked from
https://communities.vmware.com/thread/572240(Translated from german with Goolge 😉 )
-
Hmm..
With VirtualPC (Microsoft..) it was necessary, after defragmenting, to overwrite the now 'free' space with e.g. '0', only then had its dynamic reduction works.
Maybe 'thinks' VMWare that even in the actually empty areas are still data?
-
Right,
I just wanted to give the declaration as a hint. 😉
-
11 hours ago, Remy Lebeau said:The *correct* way to block the screen saver on XP and later is to use SetThreadExecutionState() with the ES_DISPLAY_REQUIRED flag:
Hmm..
and starting with W7 you have to use the
function PowerCreateRequest(REASON_CONTEXT: TREASON_CONTEXT): THandle; stdcall; external 'kernel32.dll' name 'PowerCreateRequest'; function PowerSetRequest(PowerRequestHandle: THandle; PowerRequestType : TPowerRequestType): BOOL; stdcall; external 'kernel32.dll' name 'PowerSetRequest'; function PowerClearRequest(PowerRequestHandle: THandle; PowerRequestType : TPowerRequestType): BOOL; stdcall; external 'kernel32.dll' name 'PowerClearRequest';
-
1
-
-
Hmm..
I am not using ICS, but I have a small testtool for MultiCast made with INDY 10.
It's done with D6, but you could use it for a local test...
(Indy 10 is only in the search path, not added to the zip.. 😉 )
-
1
-
-
On 4/16/2019 at 8:32 PM, Rudy Velthuis said:That is actualy the documented way to do this. I wondered why it took so long before someone came up with it.
OP probably comes from C++, where directives like override, reintroduce or overload are not necessary. If the signature is different, it overloads and does not hide.
Hmm..
I came with this some posts ago, but I think you didn't need overload when you use 'reintroduce'..
-
Hmm..
I thing 'override' is wrong, 'reintroduce' have to use! The declaration is different.
-
1
-
-
Hmm..
Take a look to ghostscript:
https://stackoverflow.com/questions/12921006/password-protected-pdf-using-ghostscript/12929181
-
1
-
-
On 4/1/2019 at 6:26 PM, dummzeuch said:Is there also a statistics about active members? Those that visited at least once and those who posted at least once in the last month?
I have got the feeling that even though the number of members is still raising, the activity is not raising but actually falling.
Hmm..
Who 'is Active'?
I login nearly every day, read nearly every changed thread..
But, of course, I only answer, if I have something to write... And this is actualy nearly nothing...
Are I am an active User (reading) or not, of course I write nearly nothing? 😉
-
1
-
-
Hmm..
I use SHOpenFolderAndSelectItems with ILCreateFromPath to open Explorer and select a file in a new Explorer window:
unit USelectFileE; interface function OpenFolderAndSelectFile(const FileName: string): boolean; implementation uses Windows, ShellAPI, ShlObj; const OFASI_EDIT = $0001; OFASI_OPENDESKTOP = $0002; {$IFDEF UNICODE} function ILCreateFromPath(pszPath: PChar): PItemIDList stdcall; external shell32 name 'ILCreateFromPathW'; {$ELSE} function ILCreateFromPath(pszPath: PChar): PItemIDList stdcall; external shell32 name 'ILCreateFromPathA'; {$ENDIF} procedure ILFree(pidl: PItemIDList) stdcall; external shell32; function SHOpenFolderAndSelectItems(pidlFolder: PItemIDList; cidl: Cardinal; apidl: pointer; dwFlags: DWORD): HRESULT; stdcall; external shell32; function OpenFolderAndSelectFile(const FileName: string): boolean; var IIDL: PItemIDList; begin result := false; IIDL := ILCreateFromPath(PChar(FileName)); if IIDL <> nil then try result := SHOpenFolderAndSelectItems(IIDL, 0, nil, 0) = S_OK; finally ILFree(IIDL); end; end; end.
-
Hmm..
the buffer for pszIconPath 'must' be have the size of MAX_PATH..
https://docs.microsoft.com/en-us/windows/desktop/api/shlobj_core/nf-shlobj_core-pickicondlg
function PickIconDlg(AHwnd : HWND; pszIconPath : PWideString; cchIconPath : DWORD; var piIconIndex : integer):integer; stdcall; external 'Shell32.dll' name 'PickIconDlg'; function PickIconDialog(var Filename: WideString; var IconIndex: Integer ): Boolean; var tmp : Array[0..MAX_PATH-1] of WideChar; // Min Size of pszIconPath must be MAX_PATH begin Result := False; FillChar(tmp[0], MAX_PATH * SizeOf(WideChar),0); Move(FileName[1],tmp[0],Length(FileName)* SizeOf(WideChar)); if ( PickIconDlg( 0, @tmp[0], MAX_PATH, IconIndex ) <> 0 ) then begin Filename := Widestring(tmp); Result := True; end; end; procedure TForm1.Button1Click(Sender: TObject); var tmpIdx : integer; tmpFile : WideString; begin tmpFile := ParamStr(0); PickIconDialog(tmpFile, tmpIdx); ShowMessage(tmpFile + ' - ' + IntToStr(tmpIdx)); end;
-
1
-
-
Hmm ..
Cockies ..
The are deleted by the browser like all tracking stuff automatically ..;)
And profiles does not work, because only a windows login ..
-
Hmm ..
When logging in always 'Remember me' is activated!
Since I'm sitting on a 'family' computer, that's unfortunate, I always have to disable it!
Is of course a matter of consideration, which is better ..
-
Hmm...
I am in the 'fluid' view.
There has been made in a previously readed thread a new post.
Click
I'm not landing on the 'first' new post but on the very first one from th Thread!Now I have to go through the whole thread and hope to remember what I read last ...
Please look, if there is a possibility as with the German DP, to put on the first new post of the thread.
Running commandline app and capturing output
in Windows API
Posted
Hmm..
I have a code here that uses pipes instead of tmp files for the output:
Keep in mind that some DOS commands only work within the CMD or give a return.
So just put 'cmd.exe /A /C' before you actually call it.
The Application.ProcessMessages(); are only needed when you ausing Memos for Output, Errors...