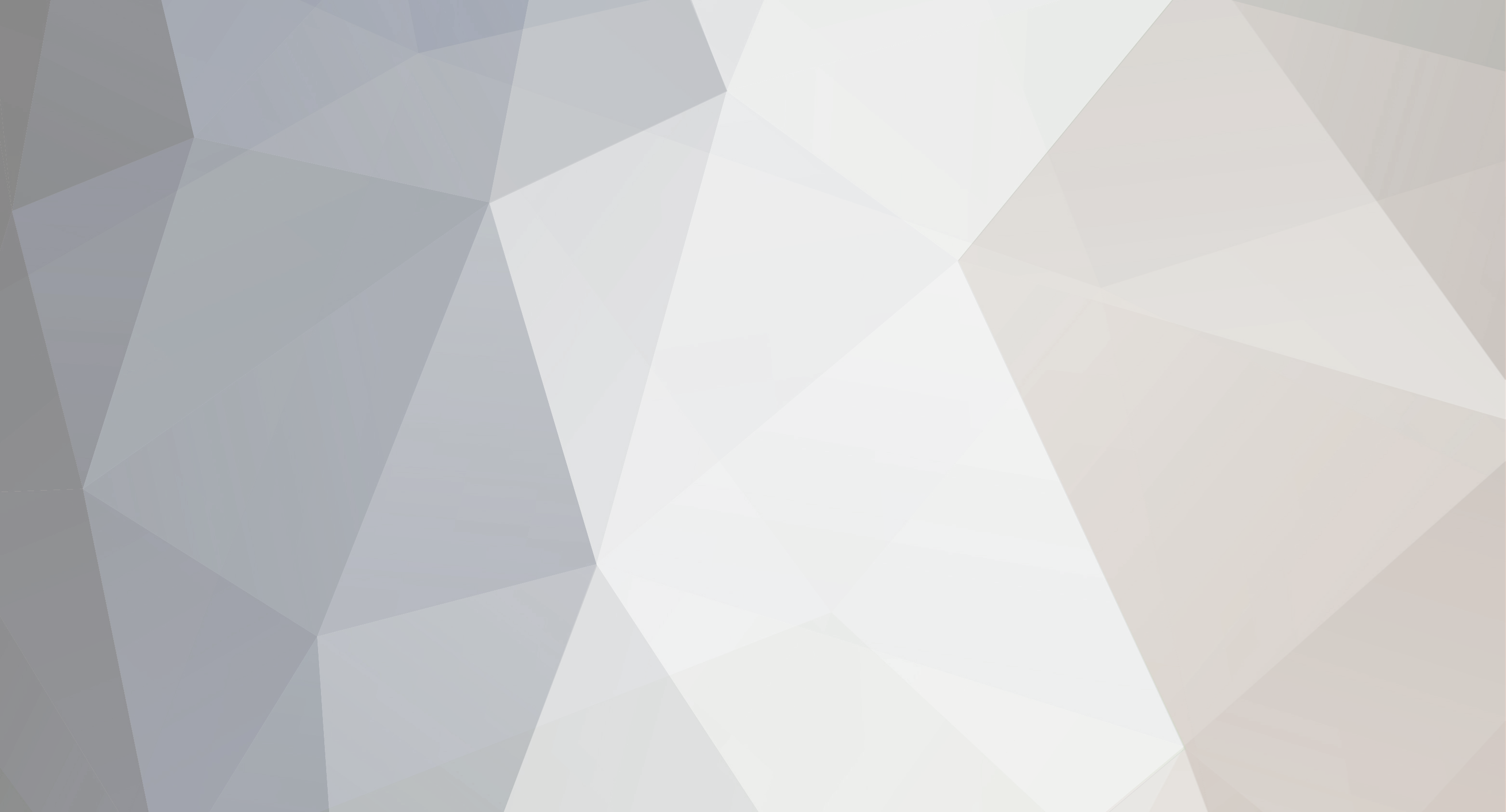
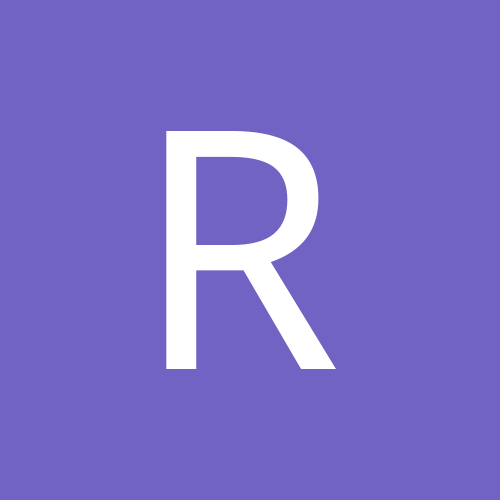
RaelB
-
Content Count
79 -
Joined
-
Last visited
Posts posted by RaelB
-
-
Which Database do you want to use?
I like Delphi-ORM. It is easy to use. It does not create the database tables.
I have a fork at https://github.com/raelb/delphi-orm - it creates the session object for SQLite without requiring a config file..
-
I'm wanting to generate a repeatable random number/character sequence based on a given seed value. Using the in built Randomize function with global RandSeed can do this, but is not thread-safe. Any recommendation for open source/light weight solution?
Thanks
-
-
-
By bad, it looks like the code does in fact execute synchronously..
-
The GUI examples work fine for demonstration purposes, but how do I use the components to execute python code and get a result within my own code, i.e synchronous execution, or be notified when execution is done?
e.g:
S := PythonExec(input_script)
Then I can use S in next line of code, or in an event that notifies script is done.
Thanks
-
Hi,
The docs explicitly state that TOmniCounter is thread safe. Is the same true with TOmniValue?
-
What does FGX stand for? 🙂
-
Thanks. So if a TForm is holding such a component, e.g. TVirtualStringTree, how can I write a method on the form, such that it will be receiving those scrolling messages from the VST?
-
Hi,
In a control with scrollbar, is it possible to detect when the user clicks down and then up on the middle scrollbar button (i.e. draggable part of the scrollbar)?
Thanks
-
Thanks, both solutions work for me.
with the TSerializeJSON, I can make it a bit simpler (since I am really after a function)
TSerializeJSON = class public class function ToJSON<T: class>(AList: TObjectList<T>) : string; end;
Then i can call without declaring a variable 🙂
-
Hi,
I create object list collections as below:
TNotes = class(TObjectList<TNote>) public ... end; TBooks = class(TObjectList<TBook>) public ... end; etc..
Is there a way I can write some generic code that will be able to take in TNotes or TBooks instances, for e.g.:
function ListToJSONString(List: TObjectList<T>): string; begin ... end;
I want to call as:
s := ListToJSONString(FNotes) or
s := ListToJSONString(FBooks)
But the function does not compile...
Can such a function be declared, or do I need to change my definition of TNotes and TBooks?
(I don't like using TObjectList<TNote> or TObjectList<TBook> in my code, since I want to create local (i.e. public) methods for each list type, and it is also simpler writing TNotes instead of TObjectList<TNote> each time)
Thanks
-
-
Is using "TJSON.ObjectToJsonObject" to convert an object into a TJSONValue (and ultimately into a string).
Is it possible for this conversion to leave out a specific field/fields, for e.g. using some type of attribute?
For example:
TPersistItem = class(TPersistent) private FChangeStatus: TChangeStatus; FId: Variant; FLoading: Boolean; procedure SetId(const Value: Variant); protected ... procedure SetChangeStatus(const Value: TChangeStatus); public ... published property ChangeStatus: TChangeStatus read FChangeStatus write SetChangeStatus; property Id: Variant read FId write SetId; end;
I don't want fields "ChangeStatus" and "Loading" to be included in the result.
Thanks
-
yes, exactly..
-
Hi,
I ask this question because I don't have 2 monitors so I can't test this for myself.
Is moving from Per Monitor V1 to Per Monitor V2 "only good and no bad", or does one need to take care of certain things when making the move?
By "only good and no bad", I mean there will be advantages, however, if certain V2 related events are not handled, the behaviour will default to V1 behaviour..
Thanks
Rael
-
Thanks for the info :).
-
On 9/17/2021 at 3:45 PM, dummzeuch said:... And not just for 10 projects but for about 50 (not all of these active at the same time, of course). That's nothing I need to imagine, that's my actual working environment.
So how do you manage working in an IDE with all those different lib versions?
- Do you use specific IDE's for specific projects?
- Are you using any tools to help you with that?..
Peter Below, once told me this:
QuoteIt is possible to create project-specific IDE configurations if you really run into performance problems and the project in question really needs only a subset of your complete component set. You can disable specific design-time packages on a per-project basis, for instance. That does not solve the long search path (and browsing path, more relevant for code insight etc.) issue, though. But you can create a copy of the IDE's registry key tree (the one under HKEY_CURRENT_USER/Software) under a new key name, modify that to only get the packages needed for the project mentioned on the IDE pathes, and start the IDE with the -r command line parameter to make it use this key instead of the default. This requires a bit of fiddling with regedit, but can pay off if you have to maintain several big projects in parallel. You would have then one shortcut to the IDE for each of the projects and use the appropriate one to load a project with the associated component set.
Perhaps you are using this idea..
-
Can one include a TDataModule with DB components in a console application?
I tried to do so, and was encountering some errors in the IDE (Delphi 10.3). Well that just might be a temporary thing in the IDE, or is this something one should not/can not do?
Thanks
-
Hello,
I have opened an old open source project in the IDE (10.3), and breakpoints seem to have been disabled for this project.
Q1. How can I re-enable breakpoints?
In "Linking" I have set "Debug Information" = True, and "Map file" = Detailed. What other setting is relevant here?
Q2. Another thing is that whenever an error occurs, the IDE goes straight to CPU view. How do I revert to the usual behaviour of going to the line of code where the error occurred?
Thanks
Rael
-
Hello,
I find when I lose my internet connection, the Delphi IDE hangs for a significant amount of time, maybe 1-2 minutes.
Is there some option(s) that I can disable so that I will not experience this?
I am on Delphi 10.3.2
Thank you
Rael
-
Ok. Recently, I have been using Dark Theme at night and Light Theme during the day, so have been switching themes quite frequently
.
-
1
-
-
-
I changed over to use https://github.com/CleverComponents/Tnef-Parser . It looks like it is a comprehensive implementation.
Invalid pointer operation when try to replace object in list
in VCL
Posted
I create a number of Frames and store them in a Frame Object List (declared as FFrames: TObjectList<TMyFrame>)
The frames are also added to the VCL form.
I try to replace a Frame with below code, but it results in an "Invalid Pointer Operation": (I'm replacing the last Frame in the list with a new one)
What's wrong with the code?
Full code follows: