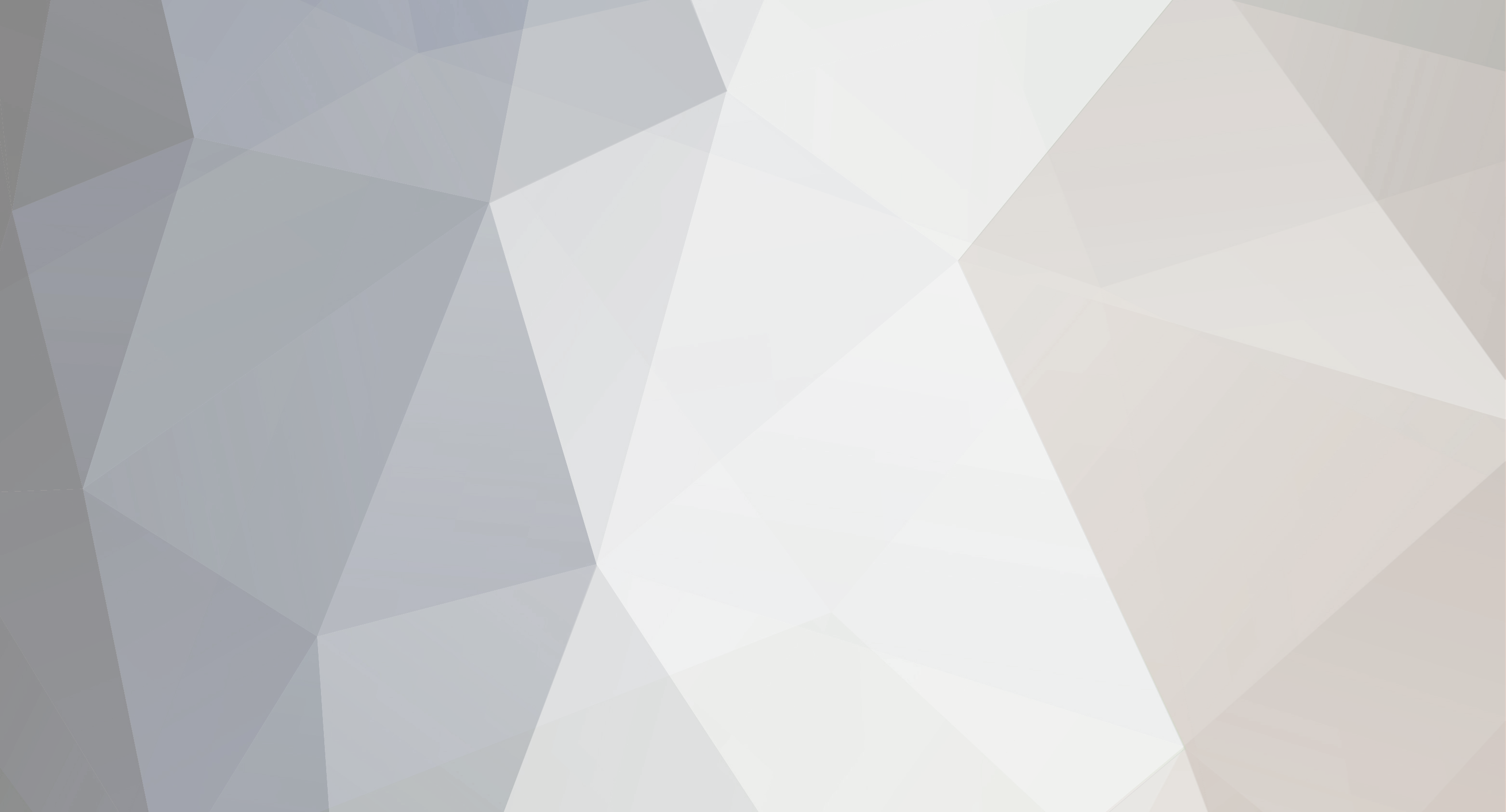
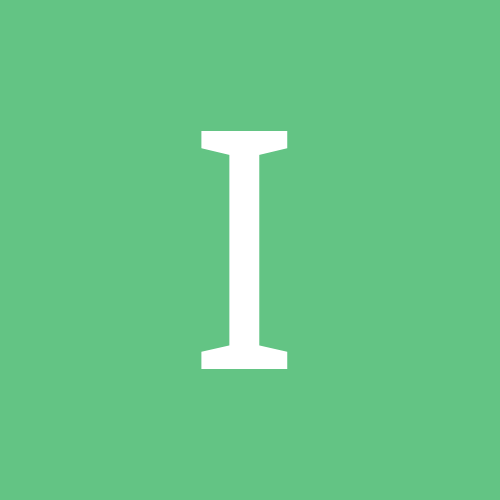
Ian Branch
-
Content Count
1435 -
Joined
-
Last visited
-
Days Won
3
Posts posted by Ian Branch
-
-
I don't have the 'Close Welcome screen when opening a new project' option checked:
-
6 minutes ago, gkobler said:Did you start Delphi in DPI Unaware Mode?
Yes. That is all I ever use.
P.S. What was your original Font style? I have changed it so many times I have forgotten.
P.P.S. I just tried the non dpi unaware version of Delphi and the Font settings all work OK.
-
-
-
Thank you for the Fonts mod.
You are right about the Treeview. 🙂
I am using a 4k monitor which runs as 125%.
I selected 10 for the font size for th Treeview and got this:
Which displayed as this:
There also seems to be a bug, perhaps resolution based, in the displaying of the .dproj names in the Controllist:
Note the indenting of the names.
No biggie, just cosmetic, all still workable.
Regards,
Ian
-
gkobler,
It would be nice to be able to change the font size.
Pretty small on a 4k monitor. 😉
-
Hi Anders,
See, I knew alternatives would be suggested. 🙂
16 minutes ago, Anders Melander said:What happens if you have two datasources connected to a single dataset
Until refresh.
20 minutes ago, Anders Melander said:support FireDAC now
I don't believe so.
20 minutes ago, Anders Melander said:What is the point of spawning a thread to update the cache if all the thread does is call Synchronize to update the cache from the main thread?
I did say it wasn't perfect... 😉
-
Hi Team,
Background:
My Pacific Island Customer is still in the 'Dark Ages' when it comes to his Network/Workstation environment. Old HP Server with Win Server 2012 r2 64bit. Two HDD in Raid 1 but one is 3Gb/s the other is 6Gb/s. 😞
His LAN workstations are old as well, running Win 7.
Users can also access my Delphi database apps on his Server via RDP and/or via the Web using Cybele's ThinFinity.The Database engine is ElevateSoft's ElevateDB 64bit.
His philosophy is, 'If it ain't broke, don't fix it.'. To which I respond, 'If it ain't as good as it can be, then it's broke'.
He just doesn't want to spend any money on it unless he absolutely has to.
Anyway, with 26+ Users and multiple multi-User Apps, things tend to bog down. Surprise!!Idea:
As he won't spend money at his end, what can I do application wise to improve the apparent database performance and therefore the User experience?
Right now you have many ideas going through your mind... 😉
What I decided, as much for the perceived need as for an exercise, was the concept of a Cacheing TDatasource. TCachedDatasource.Development:
With the assistance of two Associates, Claude & ChatGPT 4o, I developed and put the design concept/spec to them.
They each came back with a proposal which I vetted and selected one as the most promising. Also the one I could understand. 😉
So, between the three of us, playing one against the other and back, we developed a basic component that worked.
We then refined it further, eliminating some errors, optimising some areas, until I was generally satisfied and it appeared to work.
Replaced the existing TDatasource components in one of my apps with the new TCachedDatasource and tested general functionality. All good at this stage.
So, keeping this 'original' code aside, I put it to them and raised questions about various scenarios. i.e. Autoinc fields, searching, filtering, etc.
The component was slowly refined. Playing each of the AIs against each other for issues/refinements.
The overall priority was Data integrity. No loss or corruption of data. This to the detriment of any other functionality.Result:
After a day of interaction we have what is attached here.
Note some additional properties:
1. Active:- True (default) - the caching is turned on. False - The component acts like a normal TDatasource.
2. CacheSize:- This is the number of records to cache. This is associated with VisibleRecords.
3. EnableLogging:- False (default) - No logging. True - Logging enabled. A text Log will be sent to what is defined in the LogFileName property.
4. LogFileName:- Full path/name of the Log file for logging. If only a Name is entered it will be written to the Apps directory. If there is no entry here then EnableLogging is ignored.
5. RefreshInterval:- The interval, in seconds, that the cache will be refreshed. Default is 60 seconds.
6. VisibleRecords:- The number of visible records that a DBGrid may be showing. This feeds back to the CacheSize and allows dynamic adjustment if the DBGrid height is adjusted and therefore shows more, or less, records.Conclusion:
As I said, this was as much a desire to improve things for the User, as it was an exercise for myself using AI.
I'm sure it isn't perfect, and could do with some tweaks here and there. Feel free. If you find anything notable and fix it, please publish it here, I'm sure others will appreciate your submissions.
I haven't done any performance testing.This was developed in Delphi 12.2. I think it should be good back to XE2.
Learnings:
There is a lot that goes into something like this when multiple factors need to be considered.
Claude tends to forget existing code when proposing/integrating new code suggestions.
Claude was/is limited in the 'length' of Chats.
ChatGPT was reasonable but not as code particular as Claude.
Don't do this again. 🙂I hope somebody finds this of some use, even if it is as an exercise in how not to do it.
Feel free to can the whole idea. 🙂
P.S. As this was a Human/AI collaborative effort, who owns the IP?? 😉
Regards,
Ian
-
Just now, A.M. Hoornweg said:Normally Windows would open an executable file using a "memory mapped file" mechanism instead of bluntly allocating memory and loading the contents. This allows the Windows virtual memory manager to efficiently "page in" sections of the executable as needed (on demand paging), improving performance and minimizing memory use.
If the file is compressed using UPX, execution starts in the UPX loader stub which immediately allocates a big block of memory and extracts the executable into that before proceeding with the "real" execution. This simply wastes precious resources.
Interesting. I was not aware of that. Food for thought.
On what basis does UPX allocate the space/block of memory?
I guess this wouldn't really be an issue with modern Windows/PCs, but my Customer's Users are using older Win 7 SP1 PCs with 4GB of memory.
-
Hi Guys,
I acknowledge the previous two commentors and their valid inputs, however it is horses for courses. I was simply offering a suggestion.
I have used UPX for many years without any issues. Note: I use Eurekalog not MadExcept.
It provides not only compression, but also therefore a small level of obfuscation to casual file browsers.
That is all I need.
4 hours ago, Brandon Staggs said:defeats stuff the OS does to make accessing data in the file efficient
Like?
4 hours ago, Brandon Staggs said:You can zip the file for transmission bandwidth savings.
Yes I could, but, the other end is so computer illiterate that they would screw up the unzip and save process. Pacific island users. It has to be simple for them to just copy into their directory. Even then they screw it up sometimes...:-(
-
If it simply the size of the .exe you are looking to reduce, I use UPX, https://github.com/upx/upx/releases, it handles 32 & 64 bit Apps.
I use a compression value of 7 and get a roughly 30% reduction in .exe size. It will also handle wild card file names, i.e. upx -7 *.exe.
-
1
-
-
I develop on Win 11 64bit.
The question was/is about Apps created & Windows 7..
It would seem that it is all OK.
-
Hi Team,
My Customer lives in the dark ages and won't update is PCs from 32/64 bit Windows 7.
Has anybody had any issues with any of the newer Delphi language enhancements not working as desired in 32.64 bit Apps under Windows 7?
Regards & TIA,
Ian
-
Hi Anders,
Interesting. I will have a look next time. Won't be long. 😞
I have never actually had it not recover, although it has tken close to 10 minutes sometimes.
-
Hi Team,
D12.2 P2.
I have seen several comments about the IDE hanging.
Are they really sure it has?
My experience is that it appears to be hung and non-responsive but if I look in the Task Manager I can still see it doing something. No idea what.
Sometimes it takes literally minutes, very frustrating minutes, and time wasting, but it eventually returns.
I experience it most often when switching from Code to Design and vise-versa on my larger projects but from time-to-time on smaller projects as well.
Just my experience
Regards,
Ian
-
Tks Lajos,
You, that fixed it Tks. I didn't think about the race condition it would set up.
Ian
-
Hi Team,
Delphi 12.2 p2. 32 bit App.
It builds and installs OK but when I go to add it to a form Delphi thinks about it for a while, way too long, and then falls over. 😞
It is a derivation of TMonthCalendar.
Appreciate any insights.
Regards & TIA,
-
Ahhh. Thank you Uwe for the clarification and options.
Much appreciated.
-
Hi Team,
I was/am under the understanding that if I build a function library such that the DCUs are in a bin directory, add it to Delphi, and add the bin and source directories, in that order, the source should not be rebuilt with the App if an element of the library is used.
Is this the case?
The reason for the question is that this is not what is happening here.
Regards & TIA,
Ian
-
Hi Team,
I have had a small dabble with various AI machines, Meta, ChatGPT & Gemini.
None of them seem ready for prime time, yet. Getting close.
I am seeking opinions of the use of one.any of the AI's in the IDE v keeping it separate via a browser?
If in the IDE is favoured, which interface/AI?
Regards,
Ian
-
4 hours ago, Softacom | Company said:Daniel, Thomas, Lars, thank you for your continued efforts in developing this forum and keeping it alive and thriving.
Hear, Hear.
-
2
-
-
Do the DevExpress VCL components play nicely with VCL Styles?
If not, can they be made to?
Regards & TIA,
Ian
-
50 minutes ago, Jason Nelson said:64-bit IDE.... like NOBODY builds 32-bit apps anymore... unless you're a complete noob.
I build 32 bit Apps exclusively because my Customers refuse to update their 32 bit Windows 7 PCs.
Yes, I develop on a 64bit PC.
-
26 minutes ago, havrlisan said:You've got a typo: it's not System.TypeInfo, it's System.TypInfo
Doh! Well, isn't that counter intuitive...
Speaks volumes about how we 'journalise' when we read..
Thank you. All sorted now.
Regards,
Ian
wuppdi Welcome Page for Delphi 11 Alexandria?
in Delphi IDE and APIs
Posted
Sorry, I meant the actual font.