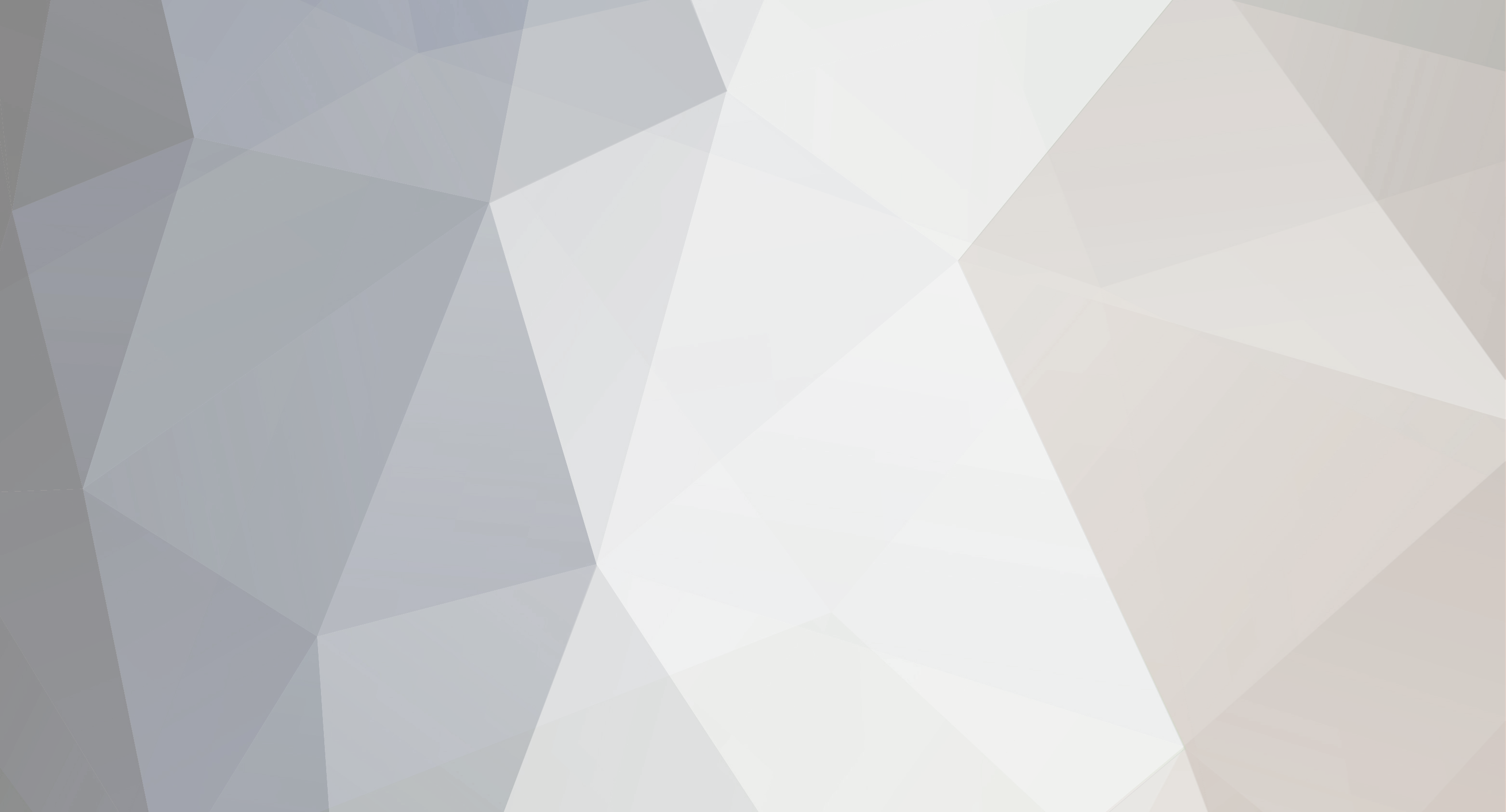
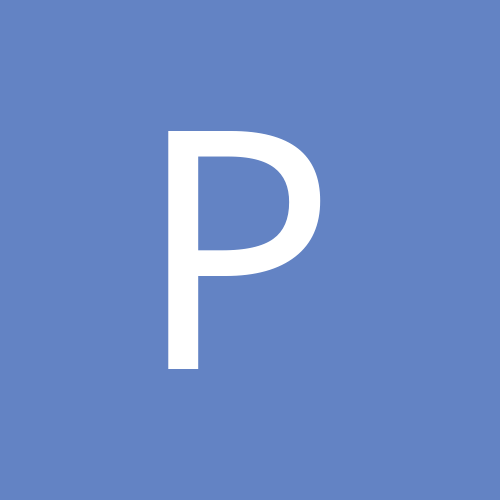
PeterBelow
Members-
Content Count
508 -
Joined
-
Last visited
-
Days Won
13
Everything posted by PeterBelow
-
Object references are not automatically managed by the compiler-generated code, unlike interface references. So your code has a couple of possible problems re lifetime control: If the assignment just replaces the RootObject.Objectlist (the internal reference held by RootObject) you leak the old object list's memory, as well as the objects held by the old list. After the assignment you now have two object list references referring to the same objectlist instance, one in RootObject.Objectlist, the other in Helper.DrawingObjectlist. If you free one the other becomes invalid and will cause an AV when next accessed. If the assignment resolves to a call to a setter method (i.e. RootObject.Objectlist is a property with a setter method) and the setter frees the old reference before storing the one passed in you do not leak memory for the old list, but you still have two references to the same object list, with the problems mentioned above. If the assignment resolves (via setter method) to clearing the content of the internal list first (assuming OwnsObject = true, so the contained objects are freed) and then storing the content of the passed list into the internal list you don't have the two problems mentioned in the first bullet point, but you have another: you now have two lists holding references to the same objects. Free an object in one list and the reference in the other becomes invalid. Especially fun if both lists have OwnsObjects set to true. If the assignment resolves (via setter method) to clearing the content of the internal list first (assuming OwnsObject = true, so the contained objects are freed) and then moving the content of the passed-in list to the internal one you don't have a memory management problem, but the source list is now empty after the assignment. That may not be acceptible, depending on your requirements. There are two ways out of this mess: Change the design from using object references to using interface references. This gives you automatic lifetime control of the objects behind the interfaces via reference counting. Implement a deep copy mechanism for the objects held in the lists. The assignment would then clear the old content of the list and then store copies (clones) of the objects in the source list into the internal list. This way the two lists are completely independent of each other, changing objects in one has no effect on the objects in the other. Both lists can have OwnsObject = true and there will be no memory leaks.
-
How to do base64 decoding then decrypt it with aes256
PeterBelow replied to Fuandi's topic in Algorithms, Data Structures and Class Design
Well, Delphi has the System.NetEncoding.TBase64Encoding class you can use for the first step to convert the string to an array of bytes (TBytes) or put it into a stream directly, e.g. a TMemorystream. The decryption is a different matter, though. There was a recent thread on using the free Lockbox 3 library for this purpose on these groups. See here... -
Application.CreateForm : Shows Main form before Application.run
PeterBelow replied to gioma's topic in VCL
Just to explain your problem: the VCL creates window handles on an as needed basis, which may delay this action until the window is shown and needs to be drawn. In your example (without Synchronize) this causes the window handle to be created in the background thread, not the main thread, and that thread has no message loop... -
64 bit shift operations?
PeterBelow replied to A.M. Hoornweg's topic in RTL and Delphi Object Pascal
Your test case is in error, your value i has all top 32 bits set to zero. -
After opening the project go to the Project Manager pane; it's docked to the right of the editor by default. Under the project node you should have a noded named "Target platforms". Right-click on it to get a menu with an "Add platform" caption. Click that to get a list of available platforms, select "Windows 64 bit" from that. You should now have two subnodes under "Target platforms", double-click on the one you want to build to activate it.
-
Do it on the string itself, like you learned addition in school. function incrementID(const aID: string):string; var ch: Char; N: Integer; begin Result := aID; N:= Length(Result); while N > 0 do begin ch := Result[N]; case ch of '0'..'8': begin Result[N] := Succ(ch); Break; end; '9': begin Result[N] := '0'; Dec(N); end; '.': Dec(N); else raise Exception.CreateFmt('Invalid character "%s" in ID "%s" at position %d.', [ch, aID, N]); end; {case ch} end; {while} end;
-
Left side cannot be assigned to
PeterBelow replied to AlanScottAgain's topic in RTL and Delphi Object Pascal
You can change the visibility of properties inherited from an ancestor, you cannot do that with fields. The VCL itself makes heavy use of that. -
EMonitorLockException help needed
PeterBelow replied to luebbe's topic in RTL and Delphi Object Pascal
As far as I can see this function does not wait at all, it just checks the task value and returns. Or does the getter for FTask.Value wait? -
Binary file encryption/decription problem?
PeterBelow replied to direktor05's topic in Algorithms, Data Structures and Class Design
Real professionals have to earn money with their works, they don't have the time to reinvent the wheel, debug and validate it. If you want to shoot yourself in the foot be my guest, but don't expect me to supply the bullets... -
Binary file encryption/decription problem?
PeterBelow replied to direktor05's topic in Algorithms, Data Structures and Class Design
Attached find a little example program that definitely works . FileEncryption.zip -
Binary file encryption/decription problem?
PeterBelow replied to direktor05's topic in Algorithms, Data Structures and Class Design
You don't need to install the component, it is just a convenience wrapper. I'll try to create a small demo over the weekend, no time at the moment. -
Make sure that all modal forms have PopupMode set to pmAuto and in the dpr file you have Application.MainformOnTaskbar := true; That avoids such isssues at least on classic Windows systems. I know nothing about Windows RD Web, though.
-
Binary file encryption/decription problem?
PeterBelow replied to direktor05's topic in Algorithms, Data Structures and Class Design
I use Lockbox 3 for such purposes. -
Delphi 11.1: DFM file completely rewritten after update
PeterBelow replied to Thomas Lassen's topic in VCL
See Tools -> Options -> User interface -> Form designer -> High DPI, the designer itself is now DPI aware and you may have to change the default setting if you design on a high DPI monitor. -
Parallels on MacOS, Designer problems
PeterBelow replied to patrik-xyndata's topic in Delphi IDE and APIs
Tools -> Options -> User interface -> Form designer -> High DPI -
Parallels on MacOS, Designer problems
PeterBelow replied to patrik-xyndata's topic in Delphi IDE and APIs
In Delphi 11 the designer itself can be configured to be DPI-aware. Which version are you using? -
IDE start randomly stops with error message
PeterBelow replied to PeterPanettone's topic in Delphi IDE and APIs
Unlikely, it would be reported much more frequently in this case. I have never seen it, for example, but in my case the only 3rd party package used is MMX... -
TStringHelper.IndexOfAny( array of string, ...): Why is this private ?
PeterBelow replied to Rollo62's topic in RTL and Delphi Object Pascal
Isn't that what it does now? -
TStringHelper.IndexOfAny( array of string, ...): Why is this private ?
PeterBelow replied to Rollo62's topic in RTL and Delphi Object Pascal
I think the core of the problem is an ambiguity inherent in the original language syntax inherited from the days of Turbo Pascal and earlier. 'x' can be interpreted either as a character constant or a string constant, and the compiler decides which it should be dependent on the context. A char is also assignment compatible with a variable or parameter of type string (but not vice versa). I see no way to get around this, and changing these rules will never happen since they make sense and changing them would break heaps of older code. Of course the compiler could use more stringent rules for overload resolution, e.g. require type identity and not only assignment compatibility. That would solve the conflict in the case you noticed but would be quite bothersome in other situations, requiring more overloaded versions of a function to be implemented. Think about what a type identity requirement would mean for overloaded functions taking numeric types as parameter. Do you really want to declare versions for byte, smallint, integer, word, cardinal etc. ? -
TStringHelper.IndexOfAny( array of string, ...): Why is this private ?
PeterBelow replied to Rollo62's topic in RTL and Delphi Object Pascal
There would probably be a conflict in overload resolution. Anyway, System.StrUtils contains IndexStr and IndexText functions that would serve your need here. -
Delphi 11.1 Stuck While Opening Project
PeterBelow replied to stacker_liew's topic in Delphi IDE and APIs
I have seen something like this occasionally (D 11.1 on Win10). Task Manager shows 100% use of one CPU core for the process. Sometimes it resolves itself after a few seconds but often the only option is to kill the process. On restarting the IDE it usually works normally. I have never been able to reproduce it at will. -
Access violation on DataModule := TDataModuleMain.Create(nil);
PeterBelow replied to ioan's topic in General Help
The VCL is not thread-safe in itsself, creating top-level objects like forms, frames or datamodules accesses some global stuff in vcl.forms connected to reading components from the dfm file. Try to replace your datamodules with a class (not derived from TCustomForm) that creates and configures all components you now place at design-time in code. I dimly remember a tool that could create such code from a dfm file, never used it myself, though. Are all your threads created in the same host thread? If so that should actually mitigate the problem since the thread constructor runs in the host thread, so all datamodules would be created in the same thread. But that may cause another problem: database connections are usually bound to the thread that creates them. If the datamodule creation already creates the connection, which is then later used in the context of the thread that owns the datamodule that may cause problems since the db access library may try to solve the conflict by serializing all DB access in a separate thread. Multithreading using a database can be even trickier than multithreading itself... -
Inline var not working this time
PeterBelow replied to Marsil's topic in RTL and Delphi Object Pascal
As far as I remember this "feature" comes from the original Wirth Pascal in Delphi's anchestry. Dropping it now would break heaps of legacy code, so is very unlikely to happen. -
How to Create dll with callback or event functions?
PeterBelow replied to ChrisChuah's topic in RTL and Delphi Object Pascal
A COM dll usually uses interfaces as callbacks as well, not function pointers. I know nothing about Docker so cannot give you any advice on this topic. .NET has an interface to unmanaged code, which is used to interact with the OS API, though. Your dll would be unmanaged code, you just have to make sure you do not use any Delphi-specific types (especially compiler-managed ones, like String or dynamic arrays) in the functions the DLL exports.