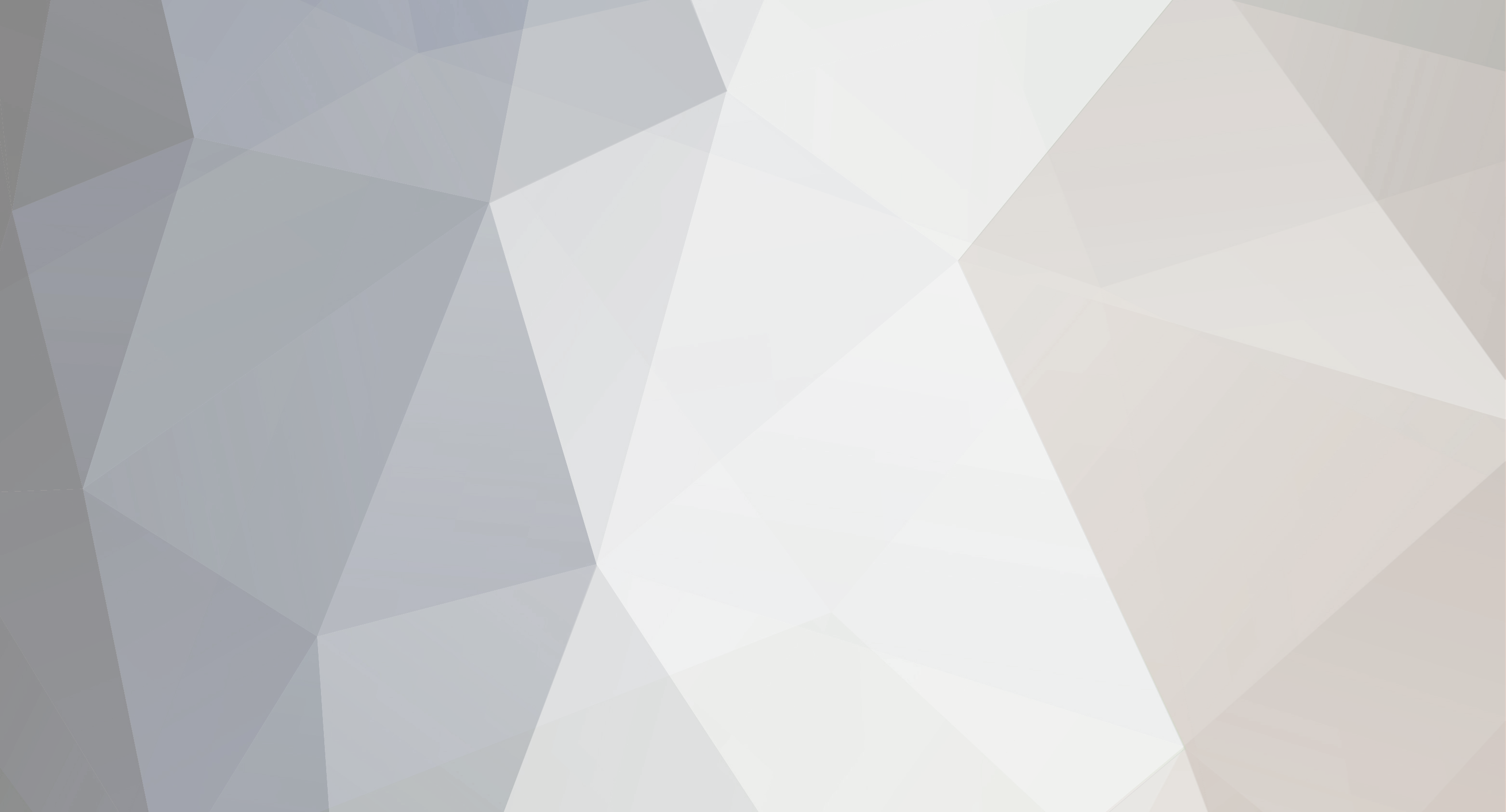
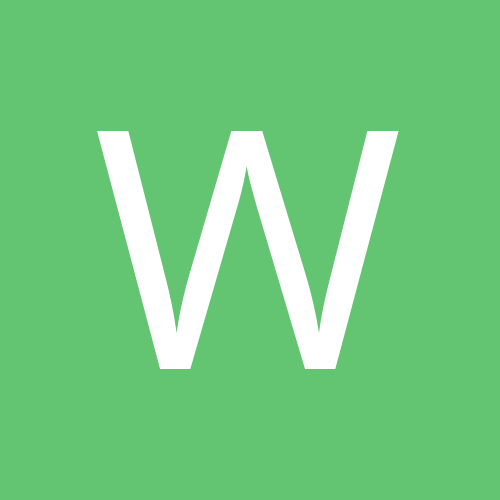
w0wbagger
Members-
Content Count
58 -
Joined
-
Last visited
-
Days Won
3
w0wbagger last won the day on May 30
w0wbagger had the most liked content!
Community Reputation
6 NeutralAbout w0wbagger
- Birthday August 11
Technical Information
-
Delphi-Version
Delphi 12 Athens
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
-
Verdict: Can confirm that if I remove the ICSPing.dcr file from the Common project file and the -k from the debug compilation additional parameters, it compiles fine in both debug and release modes, 32-bit. Will try FMX next. Then, will remove everything one more time and try the single-installation method. Edit to add: FMX installed correctly out of the box.
-
FWIW, got these three *warnings* when compiling VCLNew - not critical, but FYI: [DCC Warning] OverbyteIcsDnsQuery.pas(2248): Symbol 'StrLen' is deprecated: 'Moved to the AnsiStrings unit' [DCC Warning] OverbyteIcsDnsQuery.pas(2249): Symbol 'StrCopy' is deprecated: 'Moved to the AnsiStrings unit' [DCC Warning] OverbyteIcsMQTT.pas(1159): Comparison always evaluates to True For the last line, it's referring to this, specifically the first comparator. Because we've just done an and $03, x will always be >= 0, so: x := ReadByte (FRxStream) and $03; // V9.5 v3.1.1 may return x80 for failure if (x >= 0) and (x <= 2) then could become: x := ReadByte (FRxStream) and $03; // V9.5 v3.1.1 may return x80 for failure if x <= 2 then to eliminate the warning.
-
Hey Angus, this is in the IcsCommonCBNewDesign.cbproj file that I just downloaded from the overnights: <PropertyGroup Condition="'$(Cfg_1_Win32)'!=''"> <BCC_Defines>_DEBUG;$(BCC_Defines);$(BCC_Defines)</BCC_Defines> <BCC_UseClassicCompiler>false</BCC_UseClassicCompiler> <BCC_UserSuppliedOptions> -k</BCC_UserSuppliedOptions> <BPILibOutputDir>$(BDSCOMMONDIR)\BPL\$(Platform)\$(ProductVersion)</BPILibOutputDir> <DynamicRTL>false</DynamicRTL> <ILINK_Description>Overbyte ICS Common Design-Time Package for C++ Builder</ILINK_Description> </PropertyGroup> Also confirming that the .dcr file for ICSPing is still hanging out there alone in the CommonCBNewDesign.cbproj with no .pas file. TimeList, MimeDec, MimeUtils, and Logger correctly include the .pas in this file. Maybe your configuration ignores the spare .dcr file for some reason? Which project file should ICSPing.pas be in? common or VCL/FMX? (I haven't tested FMX yet because I don't use it, but happy to help debug it as well for other potential users). Edited to add: The difference is in whether you're doing debug or release compilation. Release has -r (which I guess is okay?), and debug has -k (which is not)
-
Roger that. I've just pulled down the overnight, am clearing all my directories, and will try again with these files.
-
I was using the overnight from 05/17/2025. ICSCommonCBNewDesign.cbproj is dated 02/10/2025 the '-k' shows up in this one. I can try to pull the most recent overnight and try again.
-
Okay! I've figured out why this was happening. There are a few units for which *only* the .dcr files are included in the icsCommonCBNewRun.cbproj file. Missing from the project file: OverbyteICSLogger.pas OverbyteICSMimeDec.pas OverbyteICSMimeUtils.pas OverbyteICSPing.pas OverbyteICSTimeList.pas In the case if OverbyteICSPing, the .dcr appears in both Common and VCL, but the .pas only appears in VCL. I don't know if it's meant to be in Common or VCL, but it probably shouldn't appear in both. For testing, I'm going to take it out of the VCL, but please let me know. I made these changes, and it compiled and installed in the 32-bit compiler. Will test with my application, but if it works it seems like we might be able to start using this new IDE! Let me know if you need further testing, after making these changes, Angus. Maybe we can get this on GetIt after all. Cheers, Ian
-
Edited to remove: LLM sent me down the wrong track.
-
Getting closer. I was able to compile icsVCLCBNewRun and icsVCLCBNewDesign after wrestling a bit with paths in the project, but it failed on installing the components with "Cannot load package 'IcsVCLCBNewRun290'. It contains 'OverbyteicsLogger', which is also contained in the package 'icsCommonCBNewDesign290'.. This is where my lack of understanding of creating/installing components is evident. Any ideas? I'll keep trying with the LLMs.
-
Solved this too: Project was not finding the icsVclCBCommonRun.bpi, which was created by that project in C:\Users\Public\Documents\Embarcadero\Studio\23.0\Dcp I added it to my global Libraries path in RAD Studio (although I could also have added it just to the project), and it compiled. I noticed that when compiling 64x, it puts the .bpi files in C:\Users\Public\Documents\Embarcadero\Studio\23.0\Dcp\Win64x, but it does *not* put the 32-bit one in C:\Users\Public\Documents\Embarcadero\Studio\23.0\Dcp\Win32 - maybe it should, and then we could add to Libraries C:\Users\Public\Documents\Embarcadero\Studio\23.0\Dcp\$(Platform) ? On to Design.
-
Understood. For my purposes, I just need to get it working in 32-bit, but it's the only component pack I use that's keeping us from moving to 64-bit, so I'll keep my eye on that once I've got 32-bit working. Thanks, Angus. My first attempt at compiling icsVclCBNewRun resulted in this: [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicstypes [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicsticks64 [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicsutils [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicscharsetutils [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicswinsock [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicsftpsrvt [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicszlibhigh [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicsstreams [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicsonetimepw [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicsmd5 [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicscrc [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicsssleay [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicswsockbuf [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicswebsession [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicsformdatadecoder [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicshtmlpars [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicsurl [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicsmimeutils [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicsntlmmsgs [ld.lld Error] ld.lld: error: undefined symbol: __init_record_Overbyteicssspi [ld.lld Error] ld.lld: error: too many errors emitted, stopping now (use /errorlimit:0 to see all errors) Investigating now.
-
For this project (ICSCommonCBNewDesign.cbproj), under options/Building/C++ Compiler/Advanced, there is an option "Additional options to pass to the compiler". There was a '-k' in there that needs to be removed. When I removed it, it compiled and installed. Moving on to VCLNewRun and VCLNewDesign.
-
Hey folks, finally have a spare weekend to attempt installing ICS v9.4 on C++ Builder v12.3, but having some issues. I just tried installing in RAD Studio 12.3 with both the April and May patches installed using C++ Builder. I was attempting to compile using the 32-bit clang (not classic) C++ Compiler. The install group did not work at all, and when I tried to install one part at a time, I was able to compile and link IcsCommonCBNewRun.cbproj, but when attempting to compile and install IcsCommonCBNewDesign.cbproj I kept getting: invalid argument 'k' Specifically, this is the command-line compiler line (see the extraneous '-k' in the end? c:\program files (x86)\embarcadero\studio\23.0\bin\bcc32c.exe -cc1 -D _DEBUG -D SECURITY_WIN32 -D USE_SSL -D MSWINDOWS -D FRAMEWORK_VCL -D USEPACKAGES -output-dir ..\Lib\Debug\Win32\23.0 -I "c:\program files (x86)\embarcadero\studio\23.0\include" -I C:\usr\Src\3rdPartyVCL\ics\Packages -I ..\Source -I ..\Lib\Debug\Win32 -isystem "c:\program files (x86)\embarcadero\studio\23.0\include" -isystem "c:\program files (x86)\embarcadero\studio\23.0\include\dinkumware64" -isystem "c:\program files (x86)\embarcadero\studio\23.0\include\windows\crtl" -isystem "c:\program files (x86)\embarcadero\studio\23.0\include\windows\sdk" -isystem "c:\program files (x86)\embarcadero\studio\23.0\include\windows\rtl" -isystem "c:\program files (x86)\embarcadero\studio\23.0\include\windows\vcl" -isystem "c:\program files (x86)\embarcadero\studio\23.0\include\windows\fmx" -isystem C:\Users\Public\Documents\Embarcadero\Studio\23.0\hpp\Win32 -isystem C:\usr\Src\3rdPartyVCL\DevExpress\VCL\Library\RS29 -isystem "C:\usr\Src\3rdPartyVCL\MyDAC for RAD Studio\Include\Win32" -isystem "C:\usr\Src\3rdPartyVCL\UniDAC for RAD Studio 12\Include\Win32" -isystem "C:\usr\Src\3rdPartyVCL\Fast Reports VCL\2025.2.2\Sources\LibRS29\VCL\Win32" -isystem C:\Users\Public\Documents\Embarcadero\Studio\23.0\hpp\Win32 -debug-info-kind=standalone -fcxx-exceptions -fborland-extensions -nobuiltininc -nostdsysteminc -triple i686-pc-windows-omf -emit-obj -mrelocation-model static -masm-verbose -ffunction-sections -fexceptions -fseh -mstack-alignment=16 -fno-spell-checking -fno-use-cxa-atexit -fno-threadsafe-statics -main-file-name IcsCommonCBNewDesign.cpp -x c++ -std=c++17 -O0 -fmath-errno -tP -tD -tM -tU -o ..\Lib\Debug\Win32\23.0\IcsCommonCBNewDesign.obj --auto-dependency-output -MT ..\Lib\Debug\Win32\23.0\IcsCommonCBNewDesign.obj -k IcsCommonCBNewDesign.cpp I'm wondering if this is a a bug in 12.3? I tried compiling this from the command line without the -k and it compiled fine. Does anyone know why it might be adding the -k? bcc32c.exe says that -k is a valid flag, but I'm wondering if that's just a holdover from the classic version. it says -k is "generate standard stack frames", but that option is set to "false" in the project option. I don't understand enough about components (at the moment, at least, trying to use ChatGPT to help me here) to get this to work easily. Would appreciate any suggestions - I'll stick with this, I can't upgrade to 12.3 until I get this working for our app. Cheers, I.
-
I just made my own __stdcall _com_issue_error(long), and it seemed to work. Now I just need to figure out why CreateInstance is failing for QBSessionManager.
-
Well, found this in utilcls.h, so I defined USING_COM_SMARTPTR, and it removed the name clashes, but even after including comdef.h, it can't find the function. Any ideas? #if defined(_INC_COMDEF) || defined(USING_COM_SMARTPTR) /* If someone wants to use definitions from COMDEF.H and UTILCLS.H, the following makes sure that the common typenames do not conflict. Define USING_COM_SMARTPTR if you have to include COMDEF.H after UTILCLS.H WARNING ======= In order to avoid name clashes (unfortunately none of these types are namespaced), we'll rename the types in UTILCLS.H to contain an '_' suffix. EXAMPLE ======= The following allows you to use both IDispatchPtr from COMDEF.H and IDispatchPtr from UTILCLS.H. IDispatchPtr msDispatch; _com_ptr version defined in COMDEF.H IDispatchPtr_ bDispatch; TCOMInterface version in UTILCLS.H */ #pragma message "Typenames collision with COMDEF.H (WARNING) " #define IDispatchPtr IDispatchPtr_ #define IUnknownPtr IUnknownPtr_ #define IFontPtr IFontPtr_ #define IFontDispPtr IFontDispPtr_ #define IPicturePtr IPicturePtr_ #define IPictureDispPtr IPictureDispPtr_ #define IEnumVARIANTPtr IEnumVARIANTPtr_ #endif
-
Hey folks, I imported the Type Library for Quickbooks Foundation Class for my C++ Builder Program (Using 12.2, 32-bit). Now, when I try to compile it, I get one of two errors. If I don't add #include "comdef.h", I get a bunch of missing _bstr_t declarations. If I *do* add "comdef.h", which is something I found recommended when getting the previous error, I get a number of typedef redefinitions (utilcls.h and comdef.h apparently define several types differently) - heres' one: c:\program files (x86)\embarcadero\studio\23.0\include\windows\sdk\comdef.h(836): error E5551: typedef redefinition with different types ('_com_ptr_t<_com_IIID<IDispatch, ((const IID *)0)> >' vs 'TComInterface< ::IDispatch, &IID_IDispatch>' (aka 'TComInterface<IDispatch, &IID_IDispatch>')) c:\program files (x86)\embarcadero\studio\23.0\include\windows\rtl\utilcls.h(2807): error E67: previous definition is here If I instead add #include "comutil.h", all the errors go away until linking, where I get: [ilink32 Error] Error: Unresolved external '__stdcall _com_issue_error(long)' referenced from C:\USR\SRC\NGB\PROIMPORT\WIN32\DEBUG\QUICKBOOKSTEST.OBJ all references to this particular unresolved external I've found online suggest adding "#include "comdef.h", which results in the *second* error up above. Maybe I can just add a #pragma link "xxxx"? What's the "xxxx" here? Thanks in advance, I.