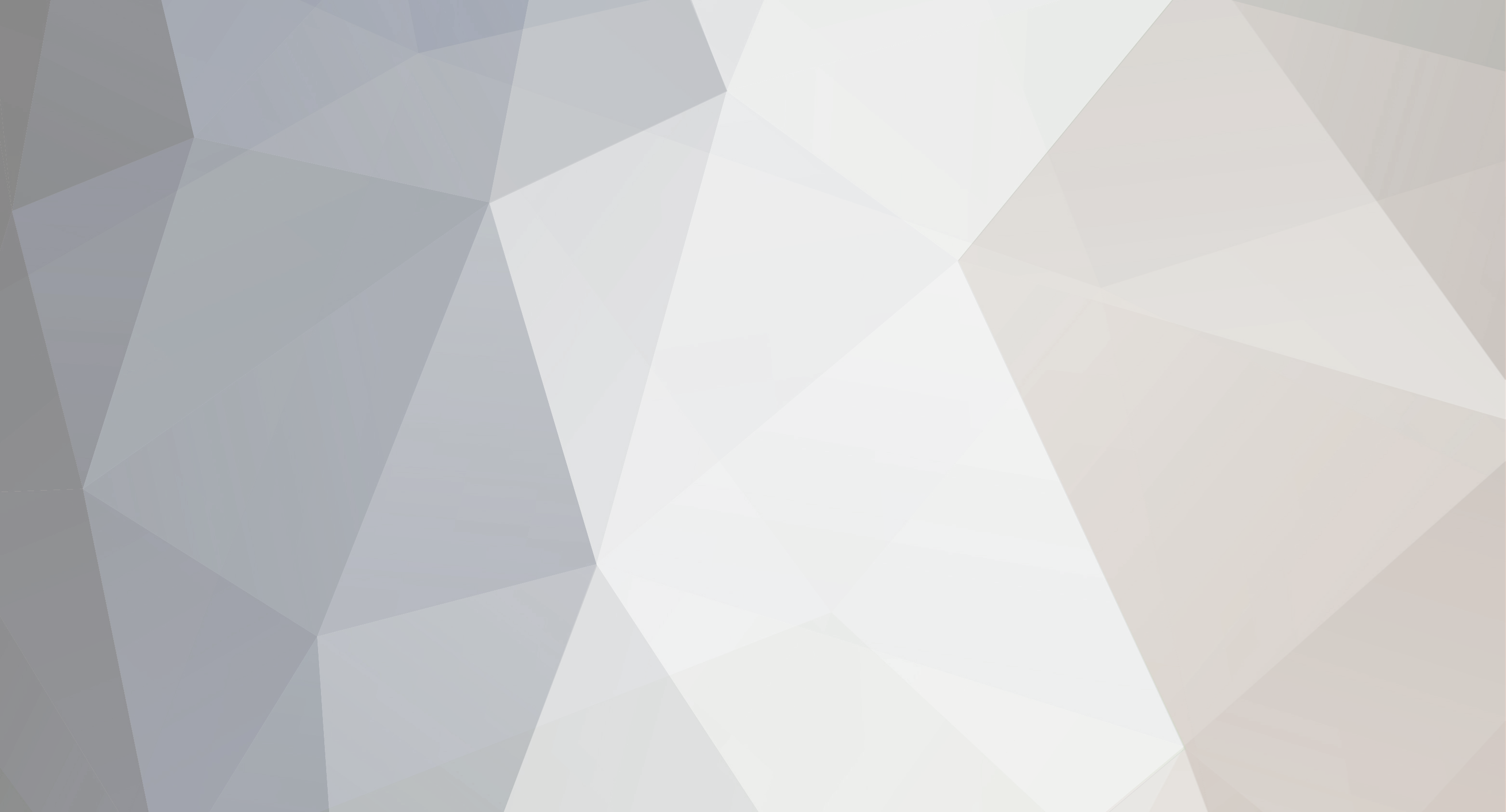
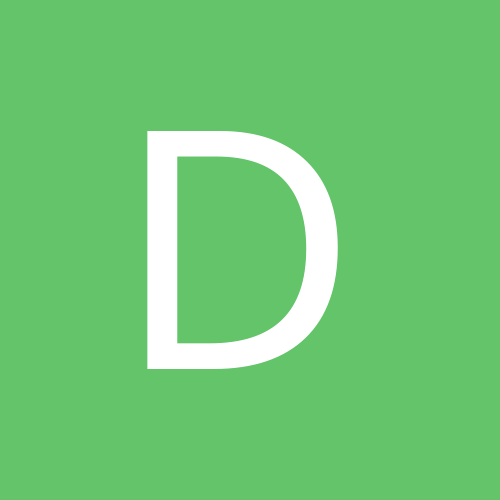
dormky
Members-
Content Count
142 -
Joined
-
Last visited
Everything posted by dormky
-
I have a TMyTable on which I've just added a field called "FileVersion" using right-click on the control, right-click again and "New Field". When I log all the fields in "MyTable.Fields.FieldName", FileVersion does indeed exist. But when setting the table's "Active" property, I get the following error : #42S22Unknown column 'FILEVERSION' in 'field list' I honestly have no idea what I'm supposed to do here ? Looking at other fields in that table, they all look the exact same. They all exist in the exact same places (in the dfm file and the code).
-
It doesn't adapt the image size to the control size. Mods, please delete this post 🙂
-
It's because with the default connection parameters, it was connecting to the production database. Which of course did not have the added column. Don't ask me why this is done by default at design time, I inherited this project...
-
Alright, so it turns out that when compiling with the DEBUG flag, all components keep the csDesigning flag in their ComponentState, leading MyDAC to connect automatically to whatever is set at design time. How can I prevent this ? Apparently SetDesigning cannot be called manually (it's protected) but of course a helper class gets around that easily. Still, I'd rather have a solution that doesn't rely on these kind of shenanigans.
-
Every field is shown in all caps in errors ; MySQL doesn't care about that anyway. And yes, I did add it in the database. And I only have one database.
-
Why is there no RTTI for arrays ?
dormky posted a topic in Algorithms, Data Structures and Class Design
TMyRecord = record myArray: array [0..1] of Integer; end; The Delphi compiler seemingly does not generate runtime type information for "myArray". This seems strange, as : TMyRecord = record myRecord: record myint: Integer; end; end; Here "myRecord" will get RTTI generated, with a name such as "TMyRecord:1". TRttiArrayType exists to represent array types, but seemingly the decision was made to just... not use it ? What's the technical reason behind the refusal to generate RTTI for this case ? It seems everything is there to do it, and the compiler just... doesn't. From an outside perpective, is seems it would be exceedingly easy to implement. -
I have a TDBRadioGroup with 2 options. Unfortunately, when pressing TAB to go from field to field, when it gets to the TDBRadioGroup you can't edit the values via keyboard. I tried space, enter and arrows, all with shift and ctrl. What's the deal here ? Is there no way to actually give the focus to this type of field and edit it via keyboard ? The TabOrder is correct and TabStop is True. Strangely enough, once you've clicked on an option it suddenly becomes available in the TAB navigation, and at the expected order. Not sure what's happening here, is it because there is no default value (none of the options are selected at first) ?
-
It's a VARCHAR(1), for the sex. The default value is empty, so none of the values are checked, which makes sense.
-
I have a build configuration that includes EurekaLog. Since EurekaLogs alone takes 15s of the build process I'd like a debug config where it's not enabled. However I can't find anywhere in the IDE allowing me to duplicate a build configuration. You can export/import options, but not entire build configurations. Is that even something possible ?
-
Ooooh I just realized you create the new config by right-clicking an existing one, not the "Build Configuration". Very cool, that works too and is much cleaner. Thanks !
-
> I assume you mean runtime warnings from custom code, and not thousands of compile time warnings? Nope, it's compile time warnings about string casts and the likes. Since a lot of the code is copy-pasted for each slightly different case, the warnings are too so there's really a lot of them ^^ The problem with creating a new configuration is that I lose the settings of the original config. We have things like a custom stack size, so I want to have the exact same config except for the Eurekalog flag and the warnings/hints. I just figured I could edit the .proj directly to make sure the configs are the exact same and it seems to be fine 🙂
-
Unfortunately, we use the Debug build to compile releases (this is probably the least problematic thing about this project lol), but there's other things I want to change in the config, such as the output of warnings (guess what when you have thousands of warnings the time spent printing it to the debug channel adds up to a lot). Also, we have so many leaks that it's not worth keeping track of at this point. More pressing things to do, somehow.
-
Why are access violations not shown in popups with EurekaLog ?
dormky posted a topic in General Help
When the program gets an access violation error, the debugger shows it fine. But when running the exe (the debug exe), it is not shown. I'm using EurekaLog and I suppose there's a setting causing this, but I can't find it. I'm using Delphi 10.3. Thanks ! -
I'd like to run this query : SELECT * FROM users WHERE id IN (:param) To get a list of the users I need. But I can't figure out how to pass an array of values to a TMyQuery object. How is this supposed to be done ? Thanks !
-
I'm not sure these answers will handle SQL sanitization properly, is there data on that ? And in any case who builds an sql accessor without giving array types lol
-
Why are access violations not shown in popups with EurekaLog ?
dormky replied to dormky's topic in General Help
No there isn't, I had to put one with a SHowMessage() in order to see it. The code is in a FormShow call. -
How do I terminate a thread that doesn't have an Execute method ?
dormky replied to dormky's topic in Algorithms, Data Structures and Class Design
That's not a problem here, the code called by the timer is "fire and forget". -
How do I terminate a thread that doesn't have an Execute method ?
dormky replied to dormky's topic in Algorithms, Data Structures and Class Design
Except that I'm putting the timer in a thread for a reason. If it's on the main thread, it runs the risk of getting delay by other work (namely, heavy drawing). If the timer is delayed, the event is delayed too. -
How do I terminate a thread that doesn't have an Execute method ?
dormky replied to dormky's topic in Algorithms, Data Structures and Class Design
Well, if someone's already made the relevant code to create a timer there's no reason to write one myself. -
How do I terminate a thread that doesn't have an Execute method ?
dormky replied to dormky's topic in Algorithms, Data Structures and Class Design
Well, it seems that no matter what I do, the timer's callback is always executed in the main thread. Anyone have a recommendation for a TTimer equivalent that has its callback executed in the thread it was created in ? -
How do I terminate a thread that doesn't have an Execute method ?
dormky replied to dormky's topic in Algorithms, Data Structures and Class Design
> You need to run your timer related code within the Execute method I would need to have the timer callback set a flag that a loop in the Execute would check ? That seems exceedingly strange. I just want a timer that exists and executes in a thread other then the main thread. The timer's interval is subject to change, and is in a class whose logic should be able to run on both main and other threads, hence why I haven't put the logic in the Execute method directly. -
How do I terminate a thread that doesn't have an Execute method ?
dormky replied to dormky's topic in Algorithms, Data Structures and Class Design
It feels so wasteful to have a method running with something like "while not Terminated do Sleep(10);". Is there not a better way of having the thread idle ? -
I've tried to install the https://github.com/CHERTS/newac component, but while it shows up in the Components list and the palette contains all the elements it should, dragging and dropping an element onto a form automatically adds the relevant units to the uses list... but Delphi can't resolve them. What could I have done wrong in the installation for this to happen ? I opened the .dpk file, right-clicked on the .bpl group and clicked Compile, then Install. Everything seemed to be fine until I actually tried it. I tried installing with both the NewAC file and the NewAC_Rio file (I'm on Delphi 10.3.2).
-
I need to make a metronome. For this purpose I've been trying to use TTimer, but it seems that for every call, it spawns a new thread. How can I prevent this and make it run in the program's main thread ? The problem is that I'm calling a windows API asynchronously, which means the timer's thread ends before the Windows call completes.