Sign in to follow this
Followers
0
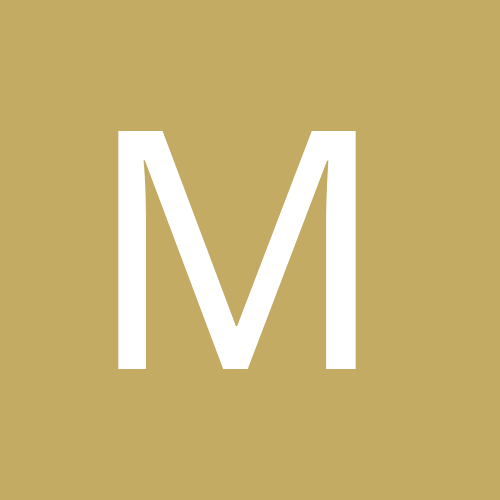
Encoding an n-dimensional array given dimensions and a list of items.
By
MarkShark, in Algorithms, Data Structures and Class Design
By
MarkShark, in Algorithms, Data Structures and Class Design