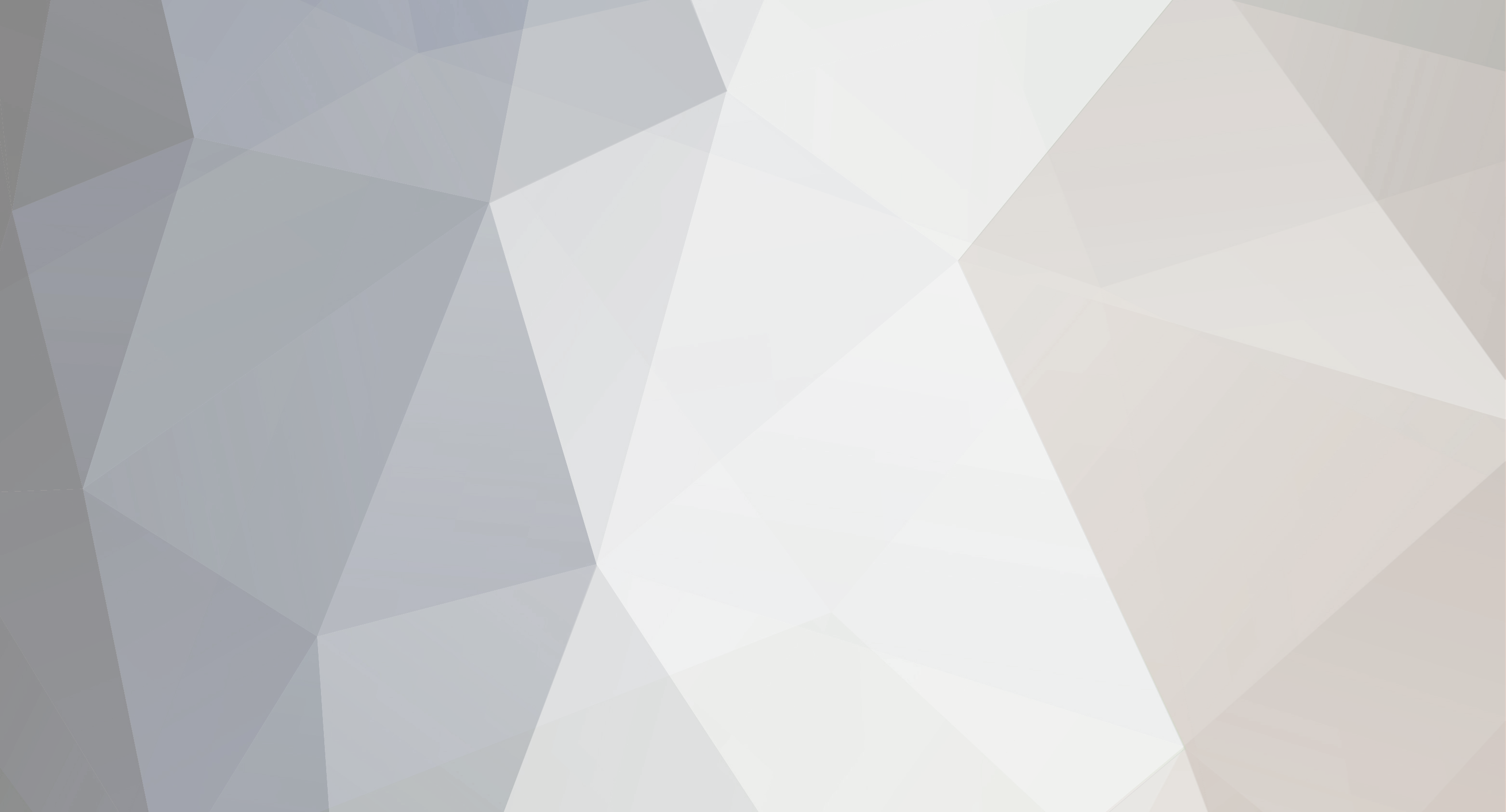
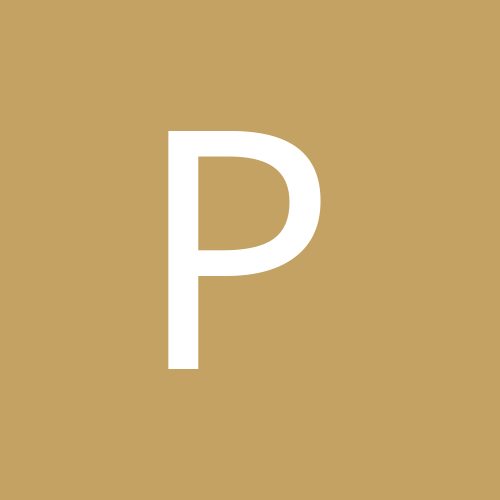
P@Sasa
-
Content Count
5 -
Joined
-
Last visited
Posts posted by P@Sasa
-
-
Maybe this would help to solve some of the problems listed above.
RAD Studio 12.2 Athens Inline Patch 1 Available
https://blogs.embarcadero.com/rad-studio-12-2-athens-inline-patch-1-available/
Unfortunately 12.2 must be uninstalled to apply the patch.
QuoteStéphane Vander ClockIt’s great to have a patch, but it’s a bit unfortunate that we have to uninstall the previous version to reinstall it with the patch. I feel quite uncomfortable with this. In the end, it’s almost the same as doing a 12.3 release. 🙁
QuoteUnderstood. We did try to get it out as a patch, but it was going to be so huge with so many files to be copied/updated that the chance for something to go wrong and leave the system partially patched and unusable was too much. In the end it made the most sense to make it an inline release to guarantee the best chance of success despite it being not quite as convenient.
-
On 9/20/2021 at 2:18 PM, Clément said:I finally got my digital certificate, and I'm signing my applications.
I'm having the same problem and thinking of getting certificate to sign EXE.
Could you please inform us what kind of certificate did you buy?
OV certificate? EV certificate? Or something else?
Thanks.
-
I also have problems with logging and here is what I did to get log file.
I make FastMM5.INC in FastMM5 directory.
{$define FastMM_EnableMemoryLeakReporting} {$define FastMM_FullDebugMode} {$define FastMM_UseOutputDebugString} {$define FastMM_ClearLogFileOnStartup} {$define FastMM_EnterDebugMode}
Then, included FastMM5.INC in FastMM5.pas before interface.
unit FastMM5; {$Include FastMM5.inc} interface
Now, when I add FastMM5 in some project I'm getting log file.
-
3
-
-
I'm working in Delphi 10.2
I will need to make Json object which would need to ignore empty integers, numbers or entire class.
For Integers I've tried with attribute [SuppressZero] and it works OK but problem is that integer output is like string, not integer.
Is there any solution for that?
Example classes:
TOtherClass = class private FStringField1: string; FIntegerField1: Integer; FDoubleField1: Double; published property StringField1: string read FStringField1 write FStringField1; property IntegerField1: Integer read FIntegerField1 write FIntegerField1; property DoubleField1: Integer read FDoubleField1 write FDoubleField1; end; TMainClass = class private FStringField: string; FIntegerField: Integer; FDoubleField: Double; FOtherClass: TOtherClass; published property StringField: string read FStringField write FStringField; property IntegerField: Integer read FIntegerField write FIntegerField; property DoubleField: Double read FDoubleField write FDoubleField; property OtherClass: TOtherClass read FOtherClass; public end;
If I fill all fields I'll get result like this:
{ "StringField": "123", "IntegerField": 1, "DoubleField": 111.11, "OtherClass": { "StringField1": "999", "IntegerField1": 9, "DoubleField1": 999.99 } }
What I need is that if integers and doubles are 0 then it shoud not exists in result, and if entire Class is empty than that class shoud not be in result Json.
That means for: Integerfield=0, DoubleField=0.00, OtherClass->StringField1='', OtherClass->IntegerField1=0, OtherClass->doublefield1=0.00 the result shoud be:
{ "StringField": "123", } not { "StringField": "123", "IntegerField": 0, "DoubleField": 0.0, "OtherClass": { "StringField1": "", "IntegerField1": 0, "DoubleField1": 0.00 } } or { "StringField": "123", "IntegerField": 0, "DoubleField": 0.0, "OtherClass": { } }
I've tried for "integer fields" something like Uwe Raabe did with Date fields and it works for integer values 0(zero) but for integers different from 0 problem is that the result in JSON is string not integer because StringConverter and StringReverter works with string.
My idea for class with attribute [SupressZero]
TOtherClass = class private FStringField1: string; [SuppressZero] FIntegerField1: Integer; [SuppressZero] FDoubleField1: Double; published property StringField1: string read FStringField1 write FStringField1; property IntegerField1: Integer read FIntegerField1 write FIntegerField1; property DoubleField1: Integer read FDoubleField1 write FDoubleField1; end; TMainClass = class private FStringField: string; [SuppressZero] FIntegerField: Integer; [SuppressZero] FDoubleField: Double; [SuppressZero] FOtherClass: TOtherClass; published property StringField: string read FStringField write FStringField; property IntegerField: Integer read FIntegerField write FIntegerField; property DoubleField: Double read FDoubleField write FDoubleField; property OtherClass: TOtherClass read FOtherClass; public end;
My code for integer fields (did not try supressZero for Double and Class because this don't work for integer fields)
type SuppressZeroAttribute = class(JsonReflectAttribute) public constructor Create; end; TSuppressZeroInterceptor = class(TJSONInterceptor) public function StringConverter(Data: TObject; Field: string): string; override; procedure StringReverter(Data: TObject; Field: string; Arg: string); override; end; implementation { SuppressZeroAttribute } constructor SuppressZeroAttribute.Create; begin inherited Create(ctString, rtString, TSuppressZeroInterceptor); end; { TSuppressZeroInterceptor } function TSuppressZeroInterceptor.StringConverter(Data: TObject; Field: string): string; var RttiContext: TRttiContext; iValue:integer; begin iValue := RttiContext.GetType(Data.ClassType).GetField(Field).GetValue(Data).AsType<Integer>; if (iValue = 0) then begin Result := EmptyStr; end else begin Result := iValue.ToString; end; end; procedure TSuppressZeroInterceptor.StringReverter(Data: TObject; Field, Arg: string); var RttiContext: TRttiContext; iValue:Integer; begin if (Arg.IsEmpty) or (Arg.StartsWith('0')) or (Arg.ToLower.Contains('null')) then begin iValue := 0; end else begin iValue := arg.ToInteger; end; RttiContext.GetType(Data.ClassType).GetField(Field).SetValue(Data, iValue); end;
If looking only string and integer fields for first class result is:
for StringField="123", IntegerField=0 { "StringField": "123" -> OK - there is no IntegerField } for StringField="123", IntegerField=123 { "StringField": "123", "IntegerField": "123" -> NOT OK - this shoud be without quotes "" }
Is there some solution for "SupressEmptyValues" for integer, double and classes that can be done with JSonReflectAttribute and TJSONInterceptor or should I look for some other solution for my problem?
Thanks for replays.
What new features would you like to see in Delphi 13?
in Delphi IDE and APIs
Posted
1. improve IDE stability (we have to remove refactoring from delphi menu (rename refactoride290.bpl to something else in BIN directory) and that is the only way we can work with some large projects without Out Of Memory violation)
2. concentrate more that current features work as intended
3. faster building (add multi threading building for one project like they did for C++)
4. repair showing of Local variables in debugger
5. decrease EXE size (32 and 64 bit versions), like in other languages where runtime versions have less MB than debug versions
6. install options for not installing anything for FireMonkey (or to separate RadStudio VCL and RadStudio FireMonkey)