Sign in to follow this
Followers
0
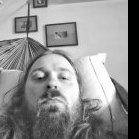
Getting RDSEED with Delphi
By
Tommi Prami, in Algorithms, Data Structures and Class Design
By
Tommi Prami, in Algorithms, Data Structures and Class Design