Sign in to follow this
Followers
0
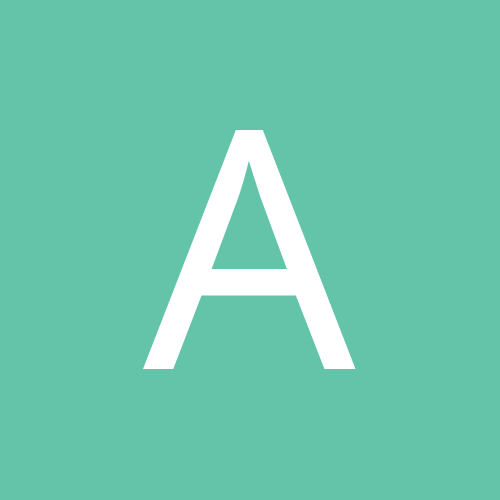
How to handle Asynchronous procedures in Delphi
By
araujoarthur, in Algorithms, Data Structures and Class Design
By
araujoarthur, in Algorithms, Data Structures and Class Design