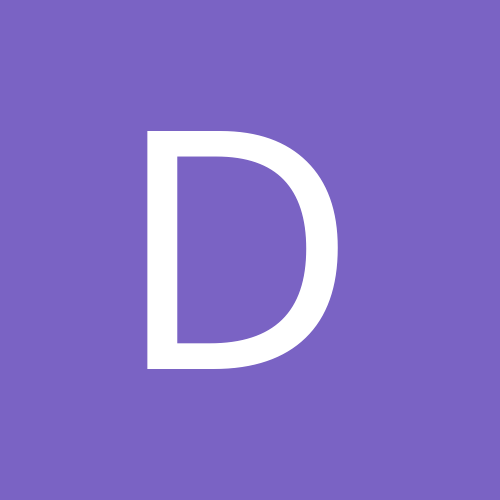
Best option for events with different parameter type in each child class?
By
Dmitry Onoshko, in Algorithms, Data Structures and Class Design
By
Dmitry Onoshko, in Algorithms, Data Structures and Class Design