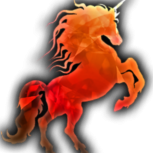
variadic-arguments How to create a Delphi variadic method similar to Write/Writeln without requiring brackets for arguments?
By
bravesofts, in Algorithms, Data Structures and Class Design
By
bravesofts, in Algorithms, Data Structures and Class Design