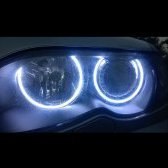
TBinaryPacket - a wrapper class to read out sent binary data over network
By
aehimself, in Algorithms, Data Structures and Class Design
By
aehimself, in Algorithms, Data Structures and Class Design