Sign in to follow this
Followers
0
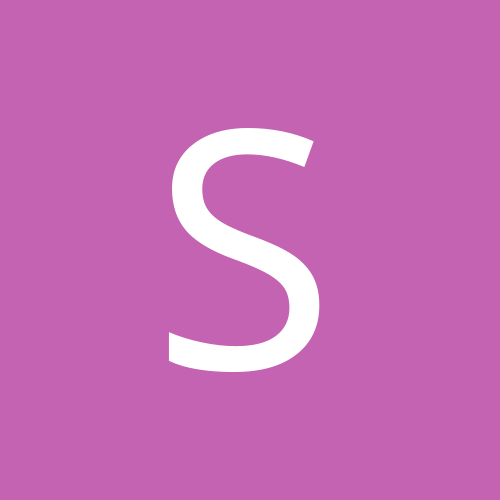
encoding pcm to opus and decoding
By
Skullcode, in Algorithms, Data Structures and Class Design
By
Skullcode, in Algorithms, Data Structures and Class Design