Sign in to follow this
Followers
0
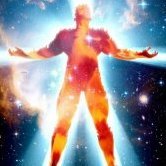
Best way to trigger in-app SoundFX supporting multiple audio devices and volume
By
Yaron, in Windows API
By
Yaron, in Windows API