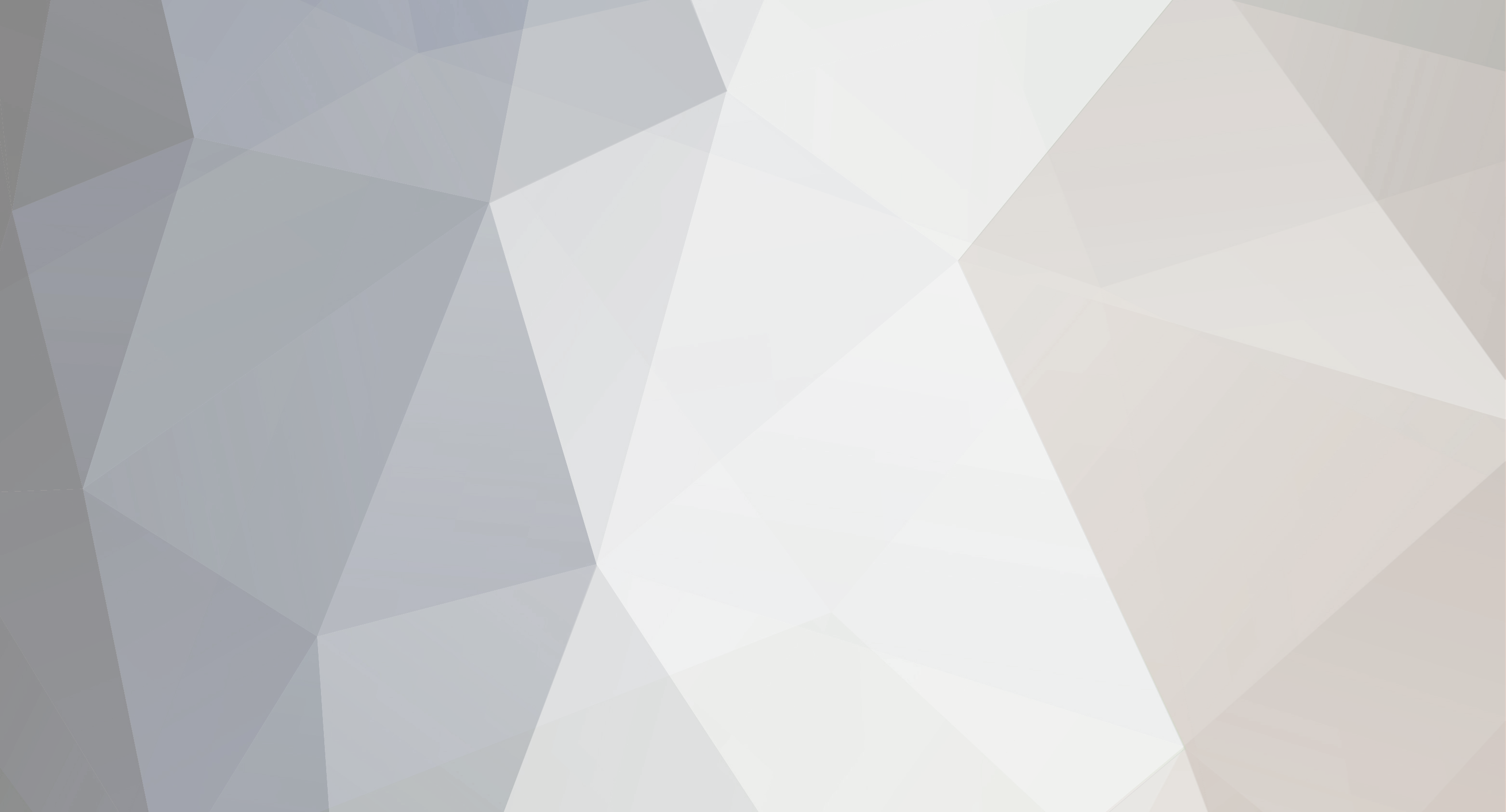
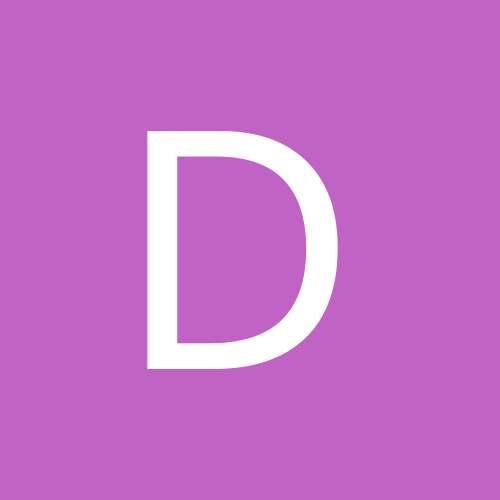
dkounal
Members-
Content Count
41 -
Joined
-
Last visited
Everything posted by dkounal
-
Revisiting TThreadedQueue and TMonitor
dkounal replied to pyscripter's topic in RTL and Delphi Object Pascal
Using TThreadedQueue<T> in Delphi 10.4.1, I have a couple of computers with windows 10 running an application of mine that have an Access violation error in line 7917 of system.generics.collections as reported by Eurekalog. it is the line: "TMonitor.Enter(FQueueLock);" It is not random. It happens all the time the first time it is used. Can you think of a reason? function TThreadedQueue<T>.PushItem(const AItem: T; var AQueueSize: Integer): TWaitResult; begin TMonitor.Enter(FQueueLock); try Result := wrSignaled; while (Result = wrSignaled) and (FQueueSize = Length(FQueue)) and not FShutDown do if not TMonitor.Wait(FQueueNotFull, FQueueLock, FPushTimeout) then Result := wrTimeout; if FShutDown or (Result <> wrSignaled) then Exit; FQueue[(FQueueOffset + FQueueSize) mod Length(FQueue)] := AItem; Inc(FQueueSize); Inc(FTotalItemsPushed); finally AQueueSize := FQueueSize; TMonitor.Exit(FQueueLock); end; TMonitor.Pulse(FQueueNotEmpty); end; -
I have to update an application that has 20 threads running at the same time and each thread is requesting an XML through an http connection. In order to process the received XML, each thread has to use data from 14 TFDMemtables. The Threads are the same running all the time and the TFDMemtables they use are also the same, The TFDMemTables' data are static, received from an external database when application starts, not need to be updated/edited as the application runs. I read that with TFDMemTable you can use clonecursor and have different TFDMemTable object instances with the same data. I though that I could create such a TFDMemTable for each thread before threads start to use them. My question: Can different threads use TFDMemTables object instances (created with data from clonecursor) from an initial TFDMemTable? Is this threadsafe? Thank you in advace
- 4 replies
-
- tfdmemtable
- tthread
-
(and 1 more)
Tagged with:
-
Return results to main thread from TTask with Thread.Queue
dkounal posted a topic in Algorithms, Data Structures and Class Design
I need to run a piece of code in TTask threads using a thread pool, that runs in every platform and get the results in the main UI thread in a thread safe message object without checking with a timer. Inspired by a post from Remy Lebeau in the Lazarus forum I wrote the following. Comments, suggestions, errors in the code welcome. interface type TTaskdata < T >= class type mydataP = ^T; mytaskproctyp =procedure(a: mydataP) of object; private Data: T; ProcessData: mytaskproctyp; procedure DoProcess; public procedure queue(datatyp: T; taskproc: mytaskproctyp); end; implementation procedure TTaskdata<T>.queue(datatyp: T; taskproc: mytaskproctyp); begin Data := datatyp; ProcessData := taskproc; TThread.queue(nil, DoProcess); end; procedure TTaskdata<T>.DoProcess; begin ProcessData(@Data); end; In an other/main Tform Unit type Dsoup = record nam: string; i: integer; end; // a sample record for send and receiving data to Ttask routine ReturnProc = procedure(mypointer: Ttaskdata<Dsoup>.mydataP) of object; var FCustomPool: TThreadPool; // the theadpool to be used for reusing threads, it should initialiazed in Tform's constructor procedure TForm1.Button1Click(Sender: TObject); // the initial route that will prepare data for Ttask and run it var soup: Dsoup; begin { set in Dsoup all data to send in TTask routine } TTask.Run(dowork(soup, getresults), FCustomPool); end; function TForm1.dowork(const a: Dsoup; p: ReturnProc): tproc; begin Result := // anonymous function to run by Ttask procedure var ff: Ttaskdata<Dsoup>; begin ff := Ttaskdata<Dsoup>.create; try { do all the task job here } ff.queue(a, p); // add result data to be sent with Thread.queue except ff.free; end; end; end; procedure TForm1.getresults(p: Ttaskdata<Dsoup>.mydataP); begin { here come the results from TTask in the main UI thread } end; -
I can not afford any more the "Can not load package" error message after installations that use DCC command line. I have a long library path with many components installed and an error like the following is presented again: warning MSB6002: The command-line for the "DCC" task is too long. Command-lines longer than 32000 characters are likely to fail. Try reducing the length of the command-line by breaking down the call to "DCC" into multiple calls with fewer parameters per call. this mean that I should remove paths from library path in the IDE and try to compile again and return them back.... a bit annoying.... Also, I hate to go to every combobox selection to add a path. The attached program reads registry and allows you to enable or disable library paths, add paths to all configs or remove from all configs. When saving it produces an .ini file with all paths (even these that are not enabled) to be used in the next time it runs No responsibility for anything, use it at your own risk, keep a backup of 'CurrentUser\SOFTWARE\Embarcadero\BDS\xxx\Library\' before using it, source at request, have a nice day! LibManager.zip
-
That's a good solution too, for many other situations. Agreed and thank you. One day, the limit arrives to this solution, too. I have 3 to 5 letters dirs, but installation programs still change path in updates and add again the path they want..... Not all the components are used at the same time. I can not afford to install and de-install them, to go behind each update to see what happened. I needed something else. Now the limit is the IDE itself.
-
I can serialize a record but I can not deserialize
dkounal posted a topic in Algorithms, Data Structures and Class Design
I need to serialize and de-serialize different records types. I wrote the following code that works ok only for the serialization and to be honest, I have not tested having records with class objects. The opposite, the de-serialization gives me an error " Internal: Cannot instantiate type .... " when calling the TJson.JsonToObject. I believe it has to do with the initialization of the new object but I can not understand the problem Any ideas? Thank you in advance The code is: type Trec4Json<T>=class private fbv:T; public class Function rec2J(a: T):string; class Function J2rec(const a: string; var c:T):boolean; property bv:T read fbv write fbv; end; implementation uses rest.json; { Trec4Json<T> } class function Trec4Json<T>.rec2J(a: T): string; // Record to Json String var b: Trec4Json<T>; begin b := Trec4Json<T>.create; b.bv := a; try result := TJson.ObjectToJsonString(b); finally b.free; end; end; class function Trec4Json<T>.J2rec(const a: string; var c: T): boolean; // Json String to Record var b: Trec4Json<T>; begin try b := TJson.JsonToObject < Trec4Json < T >> (a); c := b.bv; b.free; result := true; except result := false; end; end; -
I can serialize a record but I can not deserialize
dkounal replied to dkounal's topic in Algorithms, Data Structures and Class Design
To be honest I usually use the above rec2J to log records with the Codesite express. Now, I need to transfer different type of records in JSON through mqtt protocol. So, I was investigating the J2rec. The idea of using BSON for the mqtt seems also better. Thank you a lot -
I can serialize a record but I can not deserialize
dkounal replied to dkounal's topic in Algorithms, Data Structures and Class Design
Thank you very much. I have noticed this library in the past but I did not remember it supports records too. -
Sqlite give the possibility to create two different ':memory:' databases and attach tables from the one database to the other. Using firedac with a ':memory:' sqlite database, is it possible to attach TFDmemtables as sqlite (virtual) tables? If so, how? Thank you in advance
- 3 replies
-
- sqlite
- tfdmemtable
-
(and 1 more)
Tagged with:
-
Thank you very much for your response I have seen this documentation and I have already used TFDlocalSQL with TFDMemTables ONLY The question is: In an existing TFDConnection fot SQLite with TFDTables and TFDQueries attached to it, can I add also a TFDLocalSQL and attach TFDMemTables? I notice for example that multiple results are not supported that "If the application is using base datasets and local datasets connected to the same SQLite connection, the connection must be enabled explicitly before any dataset on this connection is opened/executed/prepared. Otherwise, an exception is raised." I am not having the Enterprise version and I am a bit blind to use it in many cases, like the TFDDatSRow object that is not explained anywhere neither in the book of Jensen. I have also find that in the above link, the examples at the end of the page goes to a Not Found http error Thank you again in advance
- 3 replies
-
- sqlite
- tfdmemtable
-
(and 1 more)
Tagged with:
-
Return results to main thread from TTask with Thread.Queue
dkounal replied to dkounal's topic in Algorithms, Data Structures and Class Design
With the last modifications, I decided to use EventBus finally. Thank you very much TigerLilly -
Thank you very much. This is really good news for my application.
- 4 replies
-
- tfdmemtable
- tthread
-
(and 1 more)
Tagged with:
-
Return results to main thread from TTask with Thread.Queue
dkounal replied to dkounal's topic in Algorithms, Data Structures and Class Design
You are correct. This was my only modification as it was reformatted completly unreadable. I give up. Please tell me what to do -
Return results to main thread from TTask with Thread.Queue
dkounal replied to dkounal's topic in Algorithms, Data Structures and Class Design
Delphi IDE 10.4.1 -> Tools -> Options -> Language ->Formatter ->select profile "Formater Default config" -> Apply. The code remains the same after Right Click->Format Source Am I missing something? Thank you in advance for your help -
Return results to main thread from TTask with Thread.Queue
dkounal replied to dkounal's topic in Algorithms, Data Structures and Class Design
I apologize. Formated using Delphi's IDE "format source" procedure -
Return results to main thread from TTask with Thread.Queue
dkounal replied to dkounal's topic in Algorithms, Data Structures and Class Design
Is it better now?