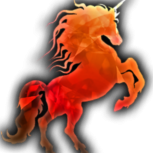
inheritance Ensuring Consistent Base Interface Implementation in Delphi Class Hierarchy
By
bravesofts, in Algorithms, Data Structures and Class Design
By
bravesofts, in Algorithms, Data Structures and Class Design