Sign in to follow this
Followers
0
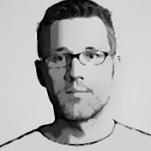
Why is TArray.BinarySearch slower than normal binary search function?
By
Mike Torrettinni, in Algorithms, Data Structures and Class Design
By
Mike Torrettinni, in Algorithms, Data Structures and Class Design