Sign in to follow this
Followers
0
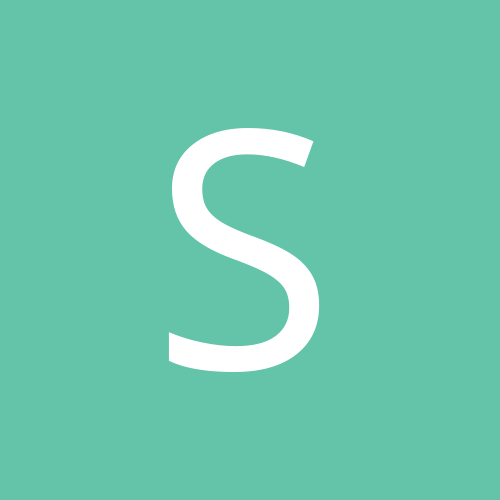
Class methods Polyformism
By
Sam Witse, in Algorithms, Data Structures and Class Design
By
Sam Witse, in Algorithms, Data Structures and Class Design