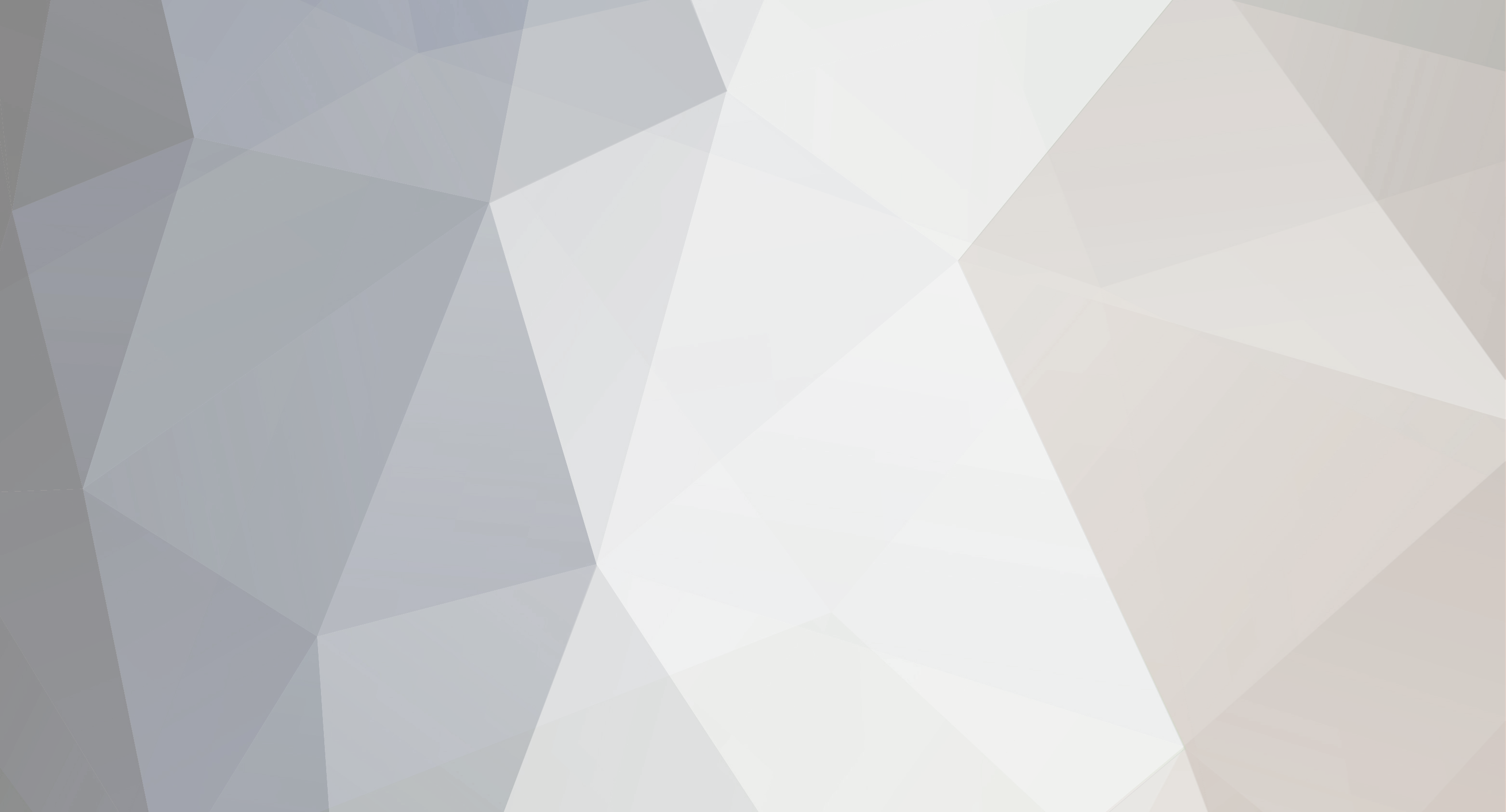
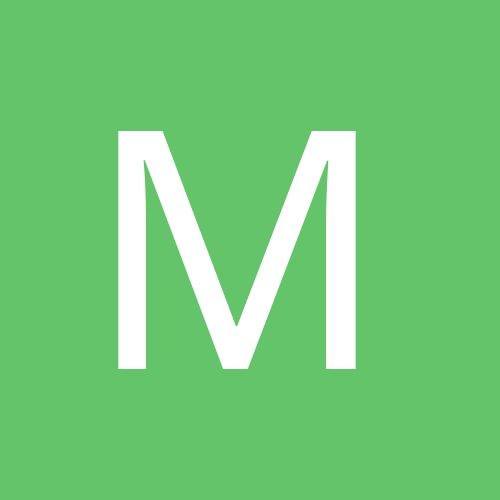
Martifan
Members-
Content Count
64 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Martifan
-
Seeing External exception 0 error using TRESTRequest on some Samsung phones
Martifan replied to Chris Pim's topic in Cross-platform
This indeed sounds like a challenging issue. A device-specific and vendor-specific problem can be tough to debug. Here are some steps you can take to troubleshoot the issue: Android Version: Check the Android version on the affected devices. It might be possible that there is some issue with a particular version of Android on these Samsung devices. Comparing Android versions between devices showing the error and those not showing the error might provide some clues. App Permissions: Make sure that the app has all necessary permissions to access the internet and any other permissions that might be needed for your app to function properly. Users might have accidentally denied some permissions. HTTP vs HTTPS: Check if the issue arises with both HTTP and HTTPS requests. Newer versions of Android have increased restrictions on plain text (non-SSL) network traffic. SSL / TLS Protocol Issues: The Samsung devices may have different SSL/TLS protocol settings. They may not support the protocol that your server uses, or there may be a bug in how the device firmware handles a particular protocol. If your server supports it, you could try switching to a different SSL/TLS protocol version to see if it resolves the issue. Network Libraries: TRESTRequest uses the platform's network libraries, which in turn use the device's firmware and potentially hardware-specific features. There might be an issue with these libraries on the affected Samsung devices. Try using an alternative HTTP library that doesn't rely on the platform's libraries (such as Indy, Synapse, etc.) to see if the issue persists. Delphi Version: You may want to try compiling your app with the latest version of Delphi. Embarcadero regularly updates their platforms to support the latest changes in each OS, so there may be a fix available if the problem is due to a Delphi RTL or FireMonkey issue. Server Side Issue: Investigate whether there's something specific about the requests coming from these Samsung devices that's causing your server to respond differently. Debugging: Since you have logcat output, even if it's not showing any errors, you can still use it for detailed debugging. Add extensive logging to your application, particularly around the network requests, and then analyze the logcat output when the problem occurs. User Community: Reach out to your user community. Ask if users experiencing the issue can provide any additional details, or if they'd be willing to help you test potential solutions. Remember, isolating the problem is half the battle in cases like this. As you gather more information and rule out potential causes, you should be able to narrow down the possibilities and get closer to a solution. Good luck! -
When dealing with FireMonkey (FMX) applications, you should remember that FMX uses a different rendering model than the VCL. In FMX, all coordinates are floating point and device-independent, and the coordinate system's origin is at the top left of the form, with positive Y coordinates going downwards. Moreover, the dimensions of the form include the non-client area (like the title bar and borders) of the form. When you set the BorderStyle property to None, you're telling FireMonkey not to include a non-client area. Therefore, FireMonkey reduces the Width and Height values to compensate for the removed non-client area. To keep the client area of the form the same, regardless of whether a non-client area is included, you need to adjust the Width and Height properties when the BorderStyle property changes. This can be done in the OnCreate event of the form. Here's an example: procedure TForm1.FormCreate(Sender: TObject); var NonClientRect: TRectF; begin BorderStyle := TFmxFormBorderStyle.None; NonClientRect := GetClientRect; // Get the client rect before changing the border style // Adjust the size Width := Width + (Width - NonClientRect.Width); Height := Height + (Height - NonClientRect.Height); end; In this code, we first get the current client rectangle before changing the border style to None. We then adjust the Width and Height of the form based on the difference between the form's original size and the client rectangle's size. This way, when you change the border style to None, the size of the client area remains the same. However, please note that you need to adjust this code if you have other components on your form that might change the size of the client area.
-
FireMonkey, the cross-platform GUI framework used in Embarcadero's RAD Studio, doesn't include the same concept of styles as, say, CSS in web development. Instead, it uses style resources and style objects, which allow you to create and apply graphical properties to various UI elements. To access and modify the current style being used by an element at runtime, you'd typically need to: Access the style object of the control (this style object is basically a mini-component tree that's used to draw the control). Once you have the style object, you can find the element you're interested in and change its properties. For example, here's some simple code to change a button's text color at runtime: procedure TForm1.Button1Click(Sender: TObject); var TextObj: TText; begin TextObj := TText(Button1.FindStyleResource('text')); if Assigned(TextObj) then TextObj.FontColor := TAlphaColorRec.Red; // Change color to red end; As you can see, we're using FindStyleResource to get the 'text' style object (which is of type TText) for the button, and then we're changing its FontColor property. Keep in mind that the names of style objects ('text' in this case) depend on the style being used and the type of control. You'll need to look at the style in the IDE's style designer to know what names to use. As for a windowborderstyle visible property, I'm not sure if there's an equivalent in FireMonkey as it has a slightly different approach to how windows and borders are managed, compared to something like Windows Forms. You might be able to use the Form.BorderStyle property to control whether or not a form has a border, but this would typically be set at design-time, not runtime. It is possible to manipulate some aspects of the window appearance at runtime using platform-specific code, but it's not as straightforward or cross-platform as most FireMonkey code. The key idea in FireMonkey is that the appearance of controls is determined by style objects, not by properties of the control itself. If you want to change how a control looks or behaves in some way, you usually need to change the style, not the control
-
[Android] How to put data from a dataset into a listbox?
Martifan replied to Fabian1648's topic in FMX
The slow speed is likely due to frequent user interface (UI) updates during the loop. To overcome this, you can use the ListBox.Items.BeginUpdate and ListBox.Items.EndUpdate methods to prevent the ListBox from updating the UI for each addition, thereby significantly speeding up the process. Here is an example of how to use it: ListBox.Items.BeginUpdate; try for i := 0 to DataList.Count - 1 do begin ... ListBoxItem.StylesData['Reference.text'] := DataList[i].Definition; ListBoxItem.StylesData['Qty1.text'] := DataList[i].Qty1; ListBoxItem.StylesData['Qty2.text'] := DataList[i].Qty2; ... end; finally ListBox.Items.EndUpdate; end; This BeginUpdate and EndUpdate pair can significantly increase the speed of your ListBox updates, as the control does not need to refresh the UI with each added item. Instead, it waits until all updates are complete and refreshes the UI just once, thus saving time. -
Yes, it is possible to get and modify style properties of a control at runtime in Delphi. To get the current style being used by a control, you can use: StyleName: string; ... StyleName := Control.StyleName; This will give you the name of the active style. Then you can get the style object itself: Style: TStyle; ... Style := StyleServices.GetStyle(StyleName); Now you can read or modify any of the style properties. For example: // Read current value BorderVisible := Style.Properties['BorderVisible']; // Modify value Style.Properties['BorderVisible'] := not BorderVisible; The TStyle has a Properties dictionary that contains all the style elements. To apply the changes to the control, call: StyleServices.SetStyle(Control, Style); So in summary: Get active style name Get TStyle object Modify properties Apply changed style This allows you to dynamically tweak the visual styles at runtime.
-
[Android] How to put data from a dataset into a listbox?
Martifan replied to Fabian1648's topic in FMX
The way you're currently doing this is creating a new TListBoxItem for each row of data, and then applying the styles and setting the data for each. This is a relatively expensive operation, particularly if you're doing it hundreds of times in a loop. Instead, consider batching your data updates. One common way to do this is to create a list of objects (each containing the three pieces of data for each row), and then update the ListBox with this list all at once. To do this, you could define a simple class to hold your data: type TListBoxData = class Definition: string; Qty1: string; Qty2: string; end; Then, you could create a list of these objects and fill it with your data: var DataList: TObjectList<TListBoxData>; i: Integer; Data: TListBoxData; begin DataList := TObjectList<TListBoxData>.Create; try for i := 0 to ... do begin Data := TListBoxData.Create; Data.Definition := value1[i]; Data.Qty1 := value2[i]; Data.Qty2 := value3[i]; DataList.Add(Data); end; UpdateListBox(DataList); finally DataList.Free; end; end; Then, in your UpdateListBox procedure, you could loop through this list of data objects and add them to the ListBox: procedure TForm1.UpdateListBox(DataList: TObjectList<TListBoxData>); var i: Integer; ListBoxItem: TListBoxItem; begin ListBox1.BeginUpdate; try for i := 0 to DataList.Count - 1 do begin ListBoxItem := TListBoxItem.Create(ListBox1); ListBoxItem.Parent := ListBox1; ListBoxItem.StylesData['Reference.text'] := DataList[i].Definition; ListBoxItem.StylesData['Qty1.text'] := DataList[i].Qty1; ListBoxItem.StylesData['Qty2.text'] := DataList[i].Qty2; ListBoxItem.ApplyStyleLookup; end; finally ListBox1.EndUpdate; end; end; This will allow you to create and set up all your TListBoxItem instances in one batch, which should significantly improve performance. If you still experience performance issues, you may want to look into using a virtualized list control that only creates and renders items as they're needed. This can significantly improve performance when dealing with large amounts of data. Please note that this code was written in Delphi since you posted Delphi-like code, but you mentioned Android in the beginning. If you're using Kotlin or Java for Android development, the approach would be different -
On mobile platforms like iOS and Android, setting the TabHeight property directly on the TabControl doesn't work as expected. The tab height is determined automatically based on the platform and controls used. Instead, to increase the tab height you need to modify the TabControl's style. Here are a couple options to try: Set the TabControl's StyleLookup property to 'tabcontrolstyle' and modify that style in the Styles Designer. Increase the height of the tab elements in the style. Clone the default TabControl style, give it a new name like 'TabControlStyleBig', and modify the tab height there. Then set your TabControl.StyleLookup to that new style name. Set the TabControl's Style property directly in code instead of using StyleLookup. Modify the Tab tabOffset and Tab tabHeight style elements. For example: TabControl1.Style.StyleElements := [seTab, seClient]; TabControl1.Style.SetElementStyle(TabControl1.Style.GetElement(seTab), [], [tsTabHeight, 70]); The key is that the tab height can't just be directly set on mobile - you have to go through the control's style. Check the documentation on TTabControlStyles and styling for more details.
-
DelphiFMX-BASS, all platforms supported by FireMonkey framework
Martifan replied to TDDung's topic in Cross-platform
That's very good, could you please show an example of how you did this? -
DelphiFMX-BASS, all platforms supported by FireMonkey framework
Martifan replied to TDDung's topic in Cross-platform
Very good, you figured out how to use audio codecs in Bass, say (Opus). I tried but there are problems with decoding -
Hello everyone, Please tell me, how do I use “Pushkit “? How do I register a “Token”? And how do I send a notification
-
UP!
-
Can you post the archive somewhere? thank you in advance
-
I have a free version, but this patch is not there
-
thanks, but it's empty, can you put it somewhere on the cloud? thank you in advance
-
where can I download it?
-
Hello, I have a problem like this: after switching from background to foreground, the application freezes for 8-10 seconds, and then continues to work and gives an error in the logs: CoreAnimation: warning, deleted thread with uncommitted CATransaction; set CA_DEBUG_TRANSACTIONS=1 in environment to log backtraces, or set CA_ASSERT_MAIN_THREAD_TRANSACTIONS=1 to abort when an implicit transaction isn't created on a main thread. what is the problem and how to solve it? thanks in advance
-
Hello Please tell me how to check the silent mode on the iPhone or not? any ideas thank you in advance
-
UP
-
Hello everyone, please tell me how to draw a route between point A to point B? on delphi, iOS
-
Delphi 10.4.1 Draws crookedly, the lines go somewhere above
-
UP
-
Hello, how can you find out what style is in the iPhone? (Black or white) P.S. Example if possible on Delphi Thanks in advance
-
oh yes, that's it, thanks the result is returned: TUserInterfaceStyle.Light или Result := TUserInterfaceStyle.Dark
-
Hello, please tell me any ideas, how can you implement authorization using Apple ID? thank you for advance
-
Thanks, it works