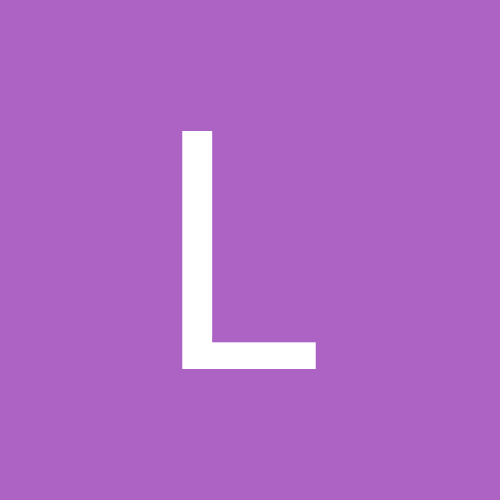
Calculation of the illumination of a point for PNG image, taking into account transparency
By
lisichkin_alexander, in Algorithms, Data Structures and Class Design
By
lisichkin_alexander, in Algorithms, Data Structures and Class Design