Sign in to follow this
Followers
0
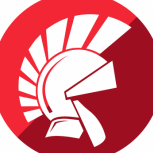
My TStringList custom SORTing, trying to mimic Windows Explorer way
By
programmerdelphi2k, in Algorithms, Data Structures and Class Design
By
programmerdelphi2k, in Algorithms, Data Structures and Class Design