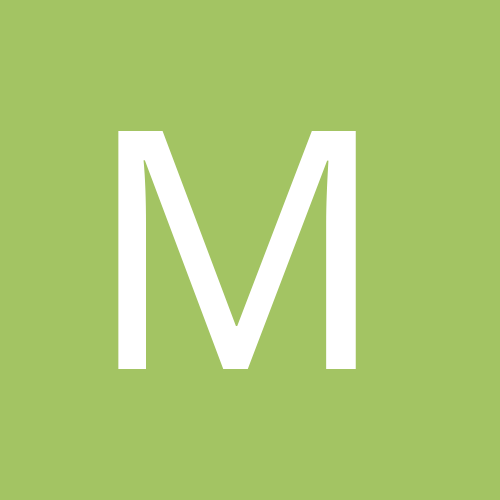
Class fields as parameters or refer to fields directly?
By
Mike Torrettinni, in Algorithms, Data Structures and Class Design
By
Mike Torrettinni, in Algorithms, Data Structures and Class Design