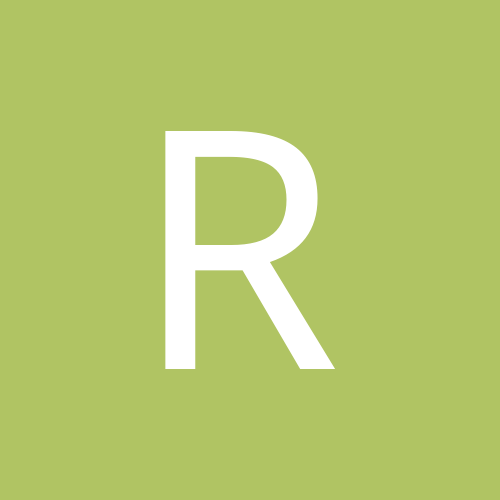
Parallel Algorithm for resampling bitmaps - very infrequent fails
By
Renate Schaaf, in Algorithms, Data Structures and Class Design
By
Renate Schaaf, in Algorithms, Data Structures and Class Design