Sign in to follow this
Followers
0
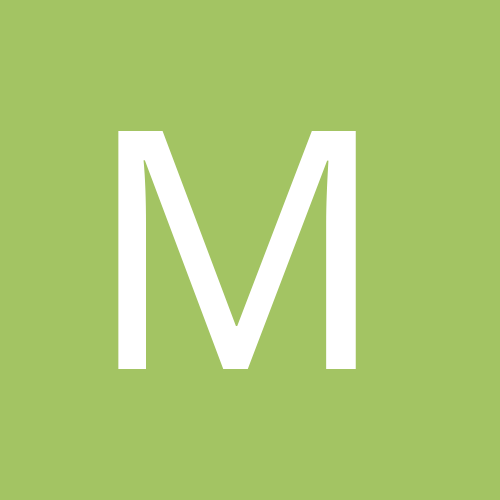
Performance - Find duplicates: Iteration vs Binary Search
By
Mike Torrettinni, in Algorithms, Data Structures and Class Design
By
Mike Torrettinni, in Algorithms, Data Structures and Class Design