Sign in to follow this
Followers
0
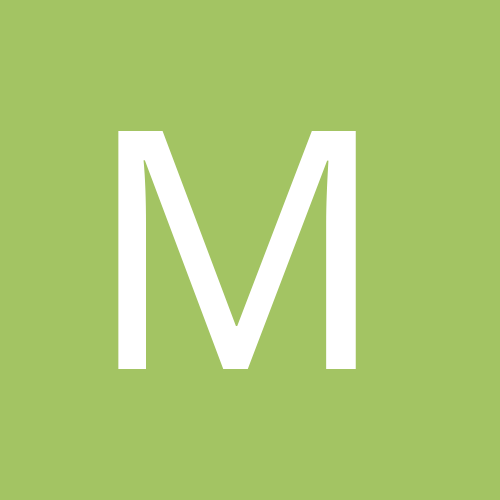
Maintaining For loop(s)
By
Mike Torrettinni, in Algorithms, Data Structures and Class Design
By
Mike Torrettinni, in Algorithms, Data Structures and Class Design