Sign in to follow this
Followers
0
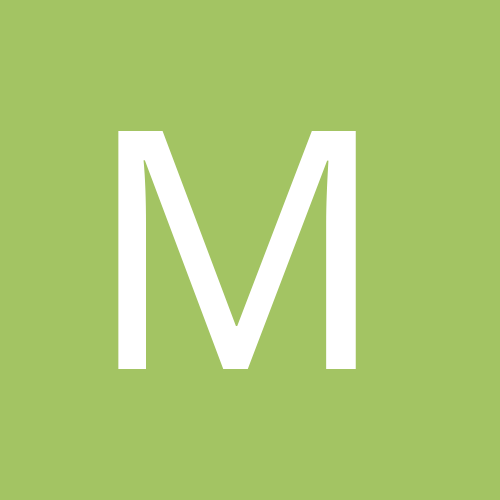
Array size increase with generics
By
Mike Torrettinni, in Algorithms, Data Structures and Class Design
By
Mike Torrettinni, in Algorithms, Data Structures and Class Design