Sign in to follow this
Followers
0
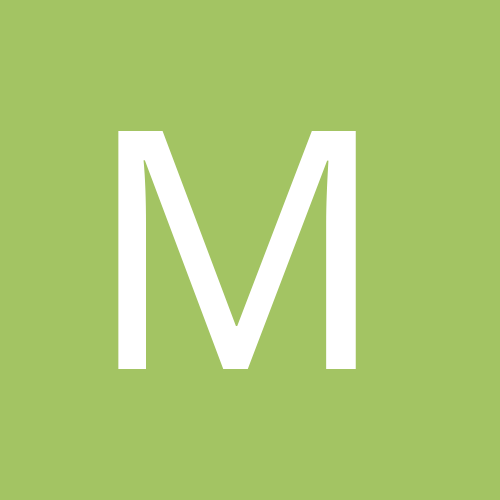
Micro optimization: use Pos before StringReplace
By
Mike Torrettinni, in Algorithms, Data Structures and Class Design
By
Mike Torrettinni, in Algorithms, Data Structures and Class Design